mybatis-plus多表分页查询代码
时间: 2023-03-13 18:30:35 浏览: 67
MyBatis-Plus提供了一种简便的方法来实现多表分页查询,可以使用Page类中的com.baomidou.mybatisplus.extension.plugins.pagination.Page对象来实现。 下面是代码示例: Page page = new Page(current, size);
List<User> users = userMapper.selectPageVo(page);
相关问题
mybatis-plus多表分页查询
可以使用Mybatis-Plus的Page对象进行多表分页查询,具体步骤如下:
1. 创建一个Page对象,设置当前页码和每页显示的记录数。
2. 使用Mybatis-Plus的PageHelper类进行分页查询,将查询结果封装到Page对象中。
3. 在Mapper接口中定义一个多表查询的方法,使用@SelectProvider注解指定SQL语句的提供者。
4. 在SQL语句的提供者中编写多表查询的SQL语句,使用Mybatis-Plus的Wrapper类进行条件查询和排序。
5. 在Mapper.xml文件中配置多表查询的SQL语句和参数映射。
6. 在Service层中调用Mapper接口的多表查询方法,获取分页查询结果。
注意:在进行多表查询时,需要注意表之间的关联关系和查询条件的设置,以确保查询结果的正确性。
mybatis-plus联表分页查询
MyBatis-Plus是一个增强版的MyBatis框架,它提供了更方便的数据库操作功能。在进行联表分页查询时,可以使用MyBatis-Plus提供的LambdaQueryWrapper和Page类来实现。
首先,确保已经准备好数据库结构以及数据,并添加了MyBatis-Plus的依赖。
然后,在配置类中启用MyBatis-Plus的join功能,可以通过在DataScopeSqlInjector类中添加@Mapper注解来实现。
接下来,在实体类中定义需要查询的字段,并生成对应的Mapper接口。
在Service类中,可以使用LambdaQueryWrapper构建查询条件,并调用mapper的selectPage方法进行分页查询。使用Page对象指定分页参数,包括当前页码和每页显示的记录数。
最后,在测试类中调用Service的方法进行查询,并打印结果。
以下是示例代码:
```java
// 实体类
@Data
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
// Mapper接口
public interface UserMapper extends BaseMapper<User> {
}
// Service类
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Override
public IPage<User> getUserListWithPage(int pageNum, int pageSize) {
Page<User> page = new Page<>(pageNum, pageSize);
LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(User::getAge, 20);
return baseMapper.selectPage(page, queryWrapper);
}
}
// 测试类
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceImplTest {
@Autowired
private UserService userService;
@Test
public void testGetUserListWithPage() {
int pageNum = 1;
int pageSize = 10;
IPage<User> userPage = userService.getUserListWithPage(pageNum, pageSize);
List<User> userList = userPage.getRecords();
for (User user : userList) {
System.out.println(user);
}
}
}
```
以上是使用MyBatis-Plus进行联表分页查询的方法。如果有任何问题,请随时提问。
相关推荐
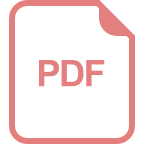
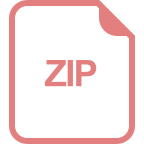
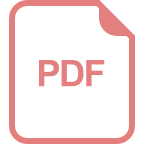












