编写函数实现(要求使用指针方法处理),计算一个字符在一个字符串中出现的次数
时间: 2023-04-04 13:02:53 浏览: 87
好的,我可以回答这个问题。以下是一个使用指针方法处理的函数,可以计算一个字符在一个字符串中出现的次数:
```c
int count_char(char *str, char c) {
int count = 0;
while (*str != '\0') {
if (*str == c) {
count++;
}
str++;
}
return count;
}
```
在这个函数中,我们使用了指针 `*str` 来遍历字符串中的每个字符。如果当前字符等于要查找的字符 `c`,则计数器 `count` 加一。最后返回计数器的值即可。
使用示例:
```c
char str[] = "hello world";
char c = 'l';
int result = count_char(str, c);
printf("'%c' appears %d times in '%s'\n", c, result, str);
```
输出:
```
'l' appears 3 times in 'hello world'
```
希望这个函数能够帮到你!
相关问题
用指针的方法编写函数实现,计算一个字符在一个字符串中出现的次数
以下是用指针的方法编写函数实现,计算一个字符在一个字符串中出现的次数的示例代码:
```c++
#include <iostream>
using namespace std;
int count_char(char* str, char ch) {
int count = 0;
while (*str != '\0') {
if (*str == ch) {
count++;
}
str++;
}
return count;
}
int main() {
char str[] = "hello world";
char ch = 'l';
int count = count_char(str, ch);
cout << "The character '" << ch << "' appears " << count << " times in the string \"" << str << "\"." << endl;
return 0;
}
```
在这个示例中,`count_char` 函数使用指针 `str` 遍历字符串中的每个字符,并使用计数器 `count` 记录字符出现的次数。`*str` 表示当前指针所指向的字符。当指针指向的字符等于给定字符 `ch` 时,计数器 `count` 加一。最后,函数返回计数器的值。
在 `main` 函数中,我们声明一个字符串 `str` 和一个字符 `ch`,并调用 `count_char` 函数计算字符 `ch` 在字符串 `str` 中出现的次数。最后,我们输出结果。
编写函数实现(要求有主函数和子函数要求使用指针方法处理),计算一个字符在一个字符串中出现的次数
好的,这是一个关于编程的问题。可以回答。以下是代码示例:
#include <stdio.h>
#include <string.h>
int countChar(char *str, char c);
int main()
{
char str[100];
char c;
printf("请输入一个字符串:");
scanf("%s", str);
printf("请输入要查找的字符:");
scanf(" %c", &c);
int count = countChar(str, c);
printf("%c 在 %s 中出现了 %d 次。\n", c, str, count);
return 0;
}
int countChar(char *str, char c)
{
int count = 0;
while (*str != '\0')
{
if (*str == c)
{
count++;
}
str++;
}
return count;
}
阅读全文
相关推荐
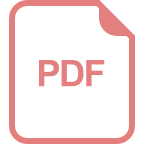
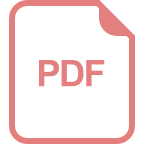
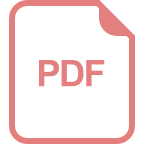













