spring boot 读取所有yml文件
时间: 2023-05-08 08:58:37 浏览: 138
在Spring Boot应用程序中,可以通过使用@ConfigurationProperties注解将配置从yml文件加载到Java类中。在默认情况下,Spring Boot将自动处理application.yml或application.yaml文件。如果想要加载其他yml文件中的配置,可以使用如下代码:
@Configuration
@PropertySource("classpath:other-config.yml")
@ConfigurationProperties(prefix = "other")
public class OtherConfig {
private String someProperty;
//其他属性
//getter和setter方法
}
在这个例子中,@PropertySource注解用来指定其他yml文件的位置,@ConfigurationProperties注解中的prefix属性指定了配置的前缀。通过这种方式,Spring Boot将会读取指定文件中针对此类的配置项,然后将这些配置项注入到此类中的属性中,方便在应用程序的其他地方使用。
当然,可以按照这种方式加载多个yml文件,只需要创建多个@ConfigurationProperties注解所在的类即可。通过这种方式,可以方便地读取多个yml文件中的配置,并在应用程序中进行统一管理和使用。
相关问题
spring boot读取yml文件
### 回答1:
在 Spring Boot 中读取 YAML 文件可以使用注解 `@Value` 或者 `@ConfigurationProperties`。
使用 `@Value` 注解:
```java
@Value("${property.name}")
private String propertyName;
```
使用 `@ConfigurationProperties` 注解:
```java
@ConfigurationProperties(prefix = "property")
@Data
public class Property {
private String name;
}
```
在 application.yml 文件中配置相应的属性:
```yml
property:
name: value
```
然后在应用程序中通过注入 Bean 的方式使用即可。
### 回答2:
Spring Boot是一个用于构建独立的、生产级别的Spring应用程序的框架,它提供了许多便利的功能,其中之一是读取yml文件。
首先,我们需要在Spring Boot项目中添加YAML文件。YAML(YAML Ain't Markup Language)是一种简洁且易读的数据序列化格式,通常用于配置文件。
在src/main/resources目录下创建一个名为application.yml的文件。在该文件中,我们可以使用键值对的形式来配置各种属性。
例如,我们可以配置服务器端口号:
server:
port: 8080
然后,我们需要创建一个类来读取yml配置文件中的属性。可以使用@ConfigurationProperties注解将该类与yml文件中的属性进行绑定。
```java
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "server")
public class ServerProperties {
private int port;
public int getPort() {
return port;
}
public void setPort(int port) {
this.port = port;
}
}
```
在上述示例中,我们使用@ConfigurationProperties注解指定了yml文件中的配置项前缀为"server",然后定义了一个整型属性port,通过对应的getter和setter方法可以获取和设置该属性的值。
最后,我们可以在其他组件或Bean中使用该ServerProperties类来获取yml文件中的属性值。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
private final ServerProperties serverProperties;
@Autowired
public MyComponent(ServerProperties serverProperties) {
this.serverProperties = serverProperties;
}
public void printServerPort() {
int port = serverProperties.getPort();
System.out.println("Server port: " + port);
}
}
```
在上述示例中,我们通过构造函数注入了ServerProperties对象,并在printServerPort方法中获取了yml文件中配置的服务器端口号,然后打印出来。
这样,我们就可以通过Spring Boot读取yml文件中的属性值了。Spring Boot会自动将yml文件中的属性与@Component注解的类进行绑定,从而方便我们使用。
Spring boot 的service类 使用yml文件的内容
Spring Boot中的`service`类通常不需要直接与YAML配置文件交互,因为它们主要是业务逻辑处理层,负责处理数据操作。然而,如果你要在Spring Boot应用中使用配置信息,比如数据库连接、API端点等,你可以将这些配置存储在一个YAML文件中,例如application.yml或application.properties。
例如,YAML配置可能会包含这样的内容:
```yaml
spring:
datasource:
url: ${DATABASE_URL}
username: ${DATABASE_USERNAME}
password: ${DATABASE_PASSWORD}
jpa:
.hibernate:
ddl-auto: update
my-service:
enabled: true
timeout: 5000ms
```
在这个例子中:
- `spring.datasource`部分配置了数据库连接的相关信息,如URL、用户名和密码,使用`${}`占位符引用环境变量。
- `spring.jpa.hibernate.ddl-auto`设置自动创建或更新数据库表。
- `my-service`是一个自定义的服务配置,如服务是否启用以及超时时间。
`service`类可以通过`@Autowired`注解从`ApplicationContext`中注入配置好的数据源或bean,而不是直接读取YAML文件。
阅读全文
相关推荐
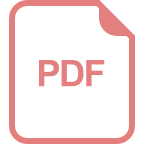
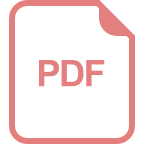
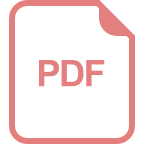
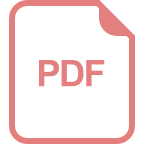
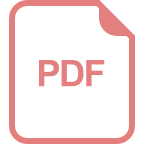
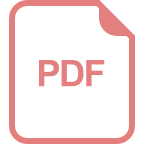
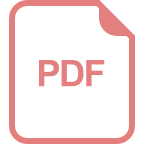
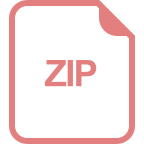
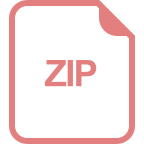
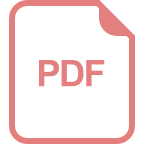
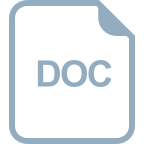





