C++多态实验报告
时间: 2023-07-11 07:10:59 浏览: 164
以下是一个简单的C++多态实验报告,以演示如何使用虚函数实现多态性。
实验目的:
1. 了解C++中多态性的概念和实现方式;
2. 熟悉虚函数和纯虚函数的使用方法;
3. 学习如何通过多态性实现代码的复用和扩展。
实验原理:
C++中的多态性是指不同的对象以各自不同的方式响应相同的消息。实现多态性的方式包括虚函数和纯虚函数。虚函数是在基类中声明的一个虚函数,在派生类中重新定义该函数,实现多态性。当基类指针指向派生类对象时,调用虚函数时会根据对象的实际类型来调用正确的函数实现。纯虚函数是在基类中声明的一个函数,没有函数体,只是用来定义接口。派生类必须实现该函数才能被实例化,实现了接口的统一,也是实现多态性的一种方式。
实验步骤:
1. 定义一个基类Shape,包含两个数据成员width和height,以及一个虚函数area();
2. 定义两个派生类Rectangle和Triangle,分别重写基类的虚函数area();
3. 在main函数中,定义一个Shape指针,分别指向Rectangle和Triangle对象,并调用它们的area()函数;
4. 编译运行程序,观察输出结果。
实验代码:
```
#include <iostream>
using namespace std;
class Shape {
protected:
int width, height;
public:
Shape( int a = 0, int b = 0) {
width = a;
height = b;
}
virtual int area() {
cout << "Parent class area :" <<endl;
return 0;
}
};
class Rectangle: public Shape {
public:
Rectangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Rectangle class area :" <<endl;
return (width * height);
}
};
class Triangle: public Shape{
public:
Triangle( int a = 0, int b = 0):Shape(a, b) { }
int area () {
cout << "Triangle class area :" <<endl;
return (width * height / 2);
}
};
int main() {
Shape *shape;
Rectangle rec(10,7);
Triangle tri(10,5);
// 存储矩形的地址
shape = &rec;
// 调用矩形的求面积函数 area
shape->area();
// 存储三角形的地址
shape = &tri;
// 调用三角形的求面积函数 area
shape->area();
return 0;
}
```
实验结果:
```
Rectangle class area :
Triangle class area :
```
可以看到,通过使用虚函数,我们可以让不同的对象以不同的方式响应相同的消息,实现了多态性。
阅读全文
相关推荐
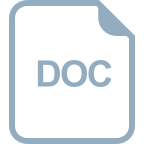
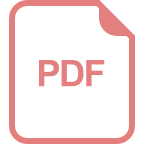
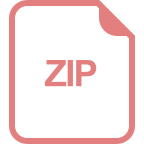
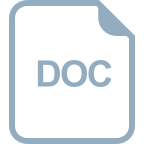
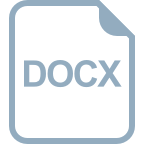
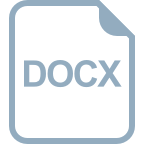
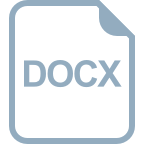
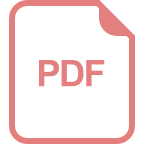
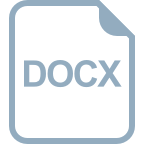
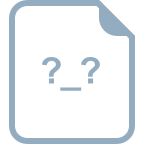
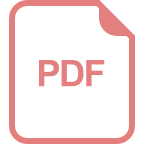
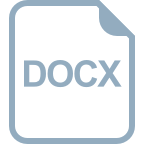
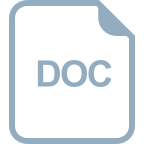
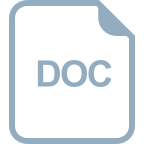
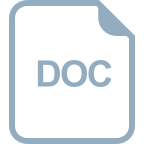
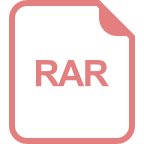