帮我构建一个使用pytorch构建的mobilenetv2网络结构
时间: 2023-02-28 10:30:45 浏览: 98
下面是使用 PyTorch 构建的 MobileNetV2 的代码示例:
```
import torch
import torch.nn as nn
class MobileNetV2(nn.Module):
def __init__(self, num_classes=1000):
super(MobileNetV2, self).__init__()
def make_divisible(v, divisor, min_value=None):
if min_value is None:
min_value = divisor
new_v = max(min_value, int(v + divisor / 2) // divisor * divisor)
if new_v < 0.9 * v:
new_v += divisor
return new_v
# building the first layer
input_channel = 32
last_channel = make_divisible(input_channel * width_mult, 8)
self.conv1 = nn.Conv2d(3, last_channel, kernel_size=3, stride=2, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(last_channel)
self.relu = nn.ReLU()
# building the inverted residual blocks
self.layer1 = self._make_layer(16, 1, last_channel)
self.layer2 = self._make_layer(24, 6, last_channel)
self.layer3 = self._make_layer(32, 6, last_channel)
self.layer4 = self._make_layer(64, 6, last_channel)
self.layer5 = self._make_layer(96, 6, last_channel)
self.layer6 = self._make_layer(160, 6, last_channel)
# building the last several layers
last_channel = make_divisible(1280 * width_mult, 8) if width_mult > 1.0 else 1280
self.conv2 = nn.Conv2d(last_channel, last_channel, kernel_size=1, stride=1, padding=0, bias=False)
self.bn2 = nn.BatchNorm2d(last_channel)
self.conv3 = nn.Conv2d(last_channel, num_classes, kernel_size=1, stride=1, padding=0, bias=False)
self.bn3 = nn.BatchNorm2d(num_classes)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
def _make_layer(self, out_channels, t, last_channel):
layers = []
for i in range(t):
layers.append(InvertedResidual(last_channel, out_channels, 2, 6))
last_channel = out_channels
return nn.Sequential(*layers)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu(x)
x = self.layer1(x)
x = self.layer2(x)
x = self
阅读全文
相关推荐
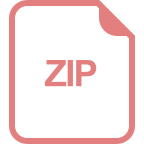
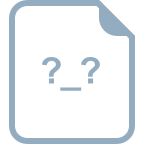
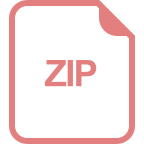
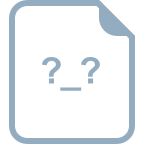
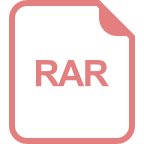
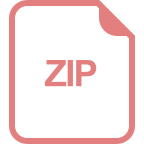
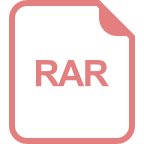
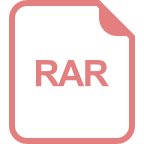
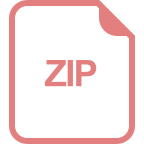
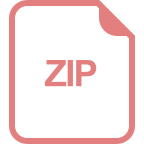
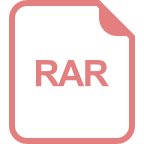
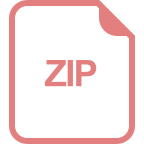
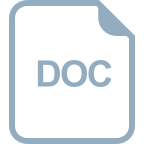
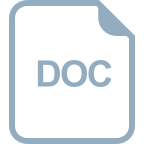
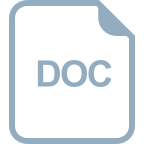

