python字符串加密解密
时间: 2023-10-21 08:06:39 浏览: 157
Python提供了多种加密解密方式,以下是其中几种常用的方法:
1. ROT13加密解密
ROT13是一种简单的加密算法,将字母表中的每个字母替换为它后面的第13个字母。例如,字母A将被替换为字母N,字母B将被替换为字母O,以此类推。ROT13加密和解密使用相同的算法。
加密代码:
```python
def rot13(message):
result = ''
for letter in message:
if letter.isalpha():
if letter.isupper():
result += chr((ord(letter) - 65 + 13) % 26 + 65)
else:
result += chr((ord(letter) - 97 + 13) % 26 + 97)
else:
result += letter
return result
```
解密代码:
```python
def rot13(message):
result = ''
for letter in message:
if letter.isalpha():
if letter.isupper():
result += chr((ord(letter) - 65 - 13) % 26 + 65)
else:
result += chr((ord(letter) - 97 - 13) % 26 + 97)
else:
result += letter
return result
```
2. Base64加密解密
Base64是一种编码方式,用于将二进制数据转换为ASCII字符,使其能够在传输过程中安全地传输。Base64编码使用64个字符来表示二进制数据,每个字符对应6个二进制位,因此它可以将3个字节的数据编码为4个字符的字符串。
加密代码:
```python
import base64
def base64_encode(message):
message_bytes = message.encode('ascii')
base64_bytes = base64.b64encode(message_bytes)
base64_message = base64_bytes.decode('ascii')
return base64_message
```
解密代码:
```python
import base64
def base64_decode(message):
base64_bytes = message.encode('ascii')
message_bytes = base64.b64decode(base64_bytes)
message = message_bytes.decode('ascii')
return message
```
3. AES加密解密
AES是一种对称加密算法,它使用相同的密钥对数据进行加密和解密。AES支持不同的密钥长度,包括128位、192位和256位。以下是使用AES加密解密字符串的示例代码:
加密代码:
```python
from Crypto.Cipher import AES
import base64
def aes_encrypt(message, key):
key = key.encode('utf-8')
message = message.encode('utf-8')
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(message)
nonce = cipher.nonce
ciphertext = base64.b64encode(ciphertext).decode('utf-8')
nonce = base64.b64encode(nonce).decode('utf-8')
tag = base64.b64encode(tag).decode('utf-8')
return ciphertext, nonce, tag
```
解密代码:
```python
from Crypto.Cipher import AES
import base64
def aes_decrypt(ciphertext, nonce, tag, key):
key = key.encode('utf-8')
ciphertext = base64.b64decode(ciphertext.encode('utf-8'))
nonce = base64.b64decode(nonce.encode('utf-8'))
tag = base64.b64decode(tag.encode('utf-8'))
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
message = cipher.decrypt_and_verify(ciphertext, tag)
message = message.decode('utf-8')
return message
```
以上是几种常用的Python字符串加密解密方法,根据实际需要选择合适的加密方式。
阅读全文
相关推荐
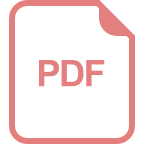
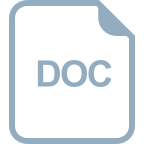
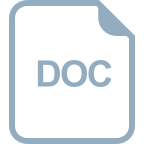
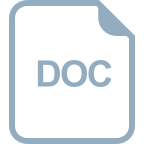






