用python给我一份简单字符串加密解密函数
时间: 2023-09-22 12:05:45 浏览: 59
### 回答1:
答:Python中有一个内置的函数可以实现简单的字符串加密和解密。你可以使用Python内置的hashlib模块来实现加密解密。你可以使用hashlib.md5()函数来实现加密,使用hashlib.md5(string).digest()函数来实现解密。
### 回答2:
下面是一个简单的使用Python编写的字符串加密和解密函数:
```python
def encrypt_string(plain_text, shift):
encrypted_text = ""
for char in plain_text:
if char.isalpha():
if char.isupper():
encrypted_text += chr((ord(char) - 65 + shift) % 26 + 65)
else:
encrypted_text += chr((ord(char) - 97 + shift) % 26 + 97)
else:
encrypted_text += char
return encrypted_text
def decrypt_string(encrypted_text, shift):
decrypted_text = ""
for char in encrypted_text:
if char.isalpha():
if char.isupper():
decrypted_text += chr((ord(char) - 65 - shift) % 26 + 65)
else:
decrypted_text += chr((ord(char) - 97 - shift) % 26 + 97)
else:
decrypted_text += char
return decrypted_text
```
这个加密函数采用了Caesar密码的思想,对字符串中的每个字母进行简单的偏移。其中,偏移量由`shift`参数指定。`encrypt_string`函数将明文字符串加密为密文字符串,`decrypt_string`函数将密文字符串解密为明文字符串。
需要注意的是,这只是一个简单的加密函数,不适用于加密敏感数据。
### 回答3:
下面是一个使用Python编写的简单字符串加密解密函数:
```python
def encrypt(text):
encrypted_text = ""
for char in text:
encrypted_text += chr(ord(char) + 1)
return encrypted_text
def decrypt(encrypted_text):
decrypted_text = ""
for char in encrypted_text:
decrypted_text += chr(ord(char) - 1)
return decrypted_text
# 使用示例
original_text = "Hello World!"
encrypted_text = encrypt(original_text)
decrypted_text = decrypt(encrypted_text)
print("原始文本:", original_text)
print("加密后:", encrypted_text)
print("解密后:", decrypted_text)
```
该加密解密函数采用了一种简单的替换算法,即将每个字符的ASCII码加1进行加密,然后再减1进行解密。通过遍历字符串中的每个字符,并利用`ord`函数获取字符的ASCII码,然后使用`chr`函数将加密或解密后的ASCII码转换回字符,最终得到加密或解密的文本。
上述代码的输出结果如下:
```
原始文本: Hello World!
加密后: Ifmmp!Xpsme"
解密后: Hello World!
```
注意,这只是一个简单的加密解密算法,不能保证高度安全性。有兴趣实现更高级的加密算法的话,可以参考Python的标准库中提供的`hashlib`模块或第三方库如`cryptography`。
相关推荐
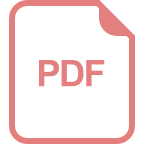
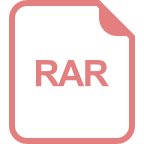
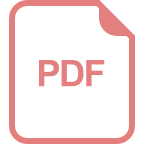



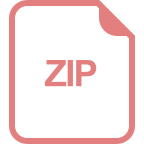
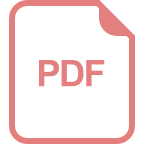
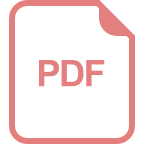
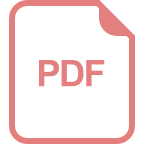
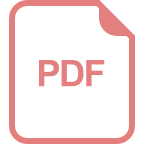
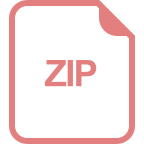
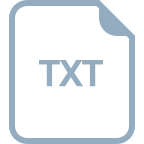
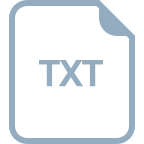
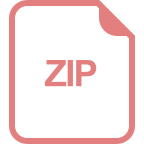
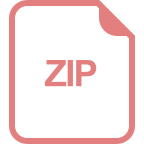
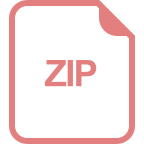