Java用两个整数栈实现一个整数队列
时间: 2024-02-11 17:40:48 浏览: 92
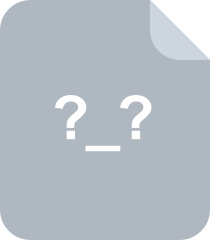
用两个栈实现一个队列的功能
Java中可以使用两个栈来实现一个整数队列。具体步骤如下:
1. 定义两个整数栈:stack1和stack2,其中stack1用于入队操作,stack2用于出队操作。
2. 入队操作:将元素压入stack1中。
3. 出队操作:先判断stack2是否为空,如果不为空,直接弹出栈顶元素;如果为空,则将stack1中的所有元素依次弹出并压入stack2中,再弹出stack2的栈顶元素。
下面是Java代码实现:
```java
import java.util.Stack;
public class MyQueue {
private Stack<Integer> stack1;
private Stack<Integer> stack2;
public MyQueue() {
stack1 = new Stack<Integer>();
stack2 = new Stack<Integer>();
}
public void push(int x) {
stack1.push(x);
}
public int pop() {
if (stack2.isEmpty()) {
while (!stack1.isEmpty()) {
stack2.push(stack1.pop());
}
}
return stack2.pop();
}
public int peek() {
if (stack2.isEmpty()) {
while (!stack1.isEmpty()) {
stack2.push(stack1.pop());
}
}
return stack2.peek();
}
public boolean empty() {
return stack1.isEmpty() && stack2.isEmpty();
}
}
```
这样,我们就用两个整数栈实现了一个整数队列。
阅读全文
相关推荐



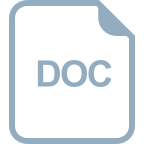
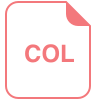
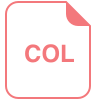
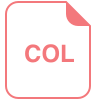





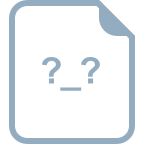