用c++写类的通讯录管理系统包含添加联系人、显示联系人、删除联系人、查找联系人、修改联系人、清空联系人、退出通讯录功能
时间: 2023-07-10 14:37:27 浏览: 49
好的,我可以帮你设计一个基于C++的通讯录管理系统。首先,我们需要定义一个 Contact 类来表示每个联系人的信息,如姓名、电话号码、地址等。然后我们可以定义一个 ContactBook 类来管理所有联系人。
以下是一个可能的实现方式:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 定义联系人类
class Contact {
public:
Contact(string name, string phone, string address)
: name_(name), phone_(phone), address_(address) {}
string name() const { return name_; }
string phone() const { return phone_; }
string address() const { return address_; }
void set_name(string name) { name_ = name; }
void set_phone(string phone) { phone_ = phone; }
void set_address(string address) { address_ = address; }
private:
string name_;
string phone_;
string address_;
};
// 定义通讯录类
class ContactBook {
public:
// 添加联系人
void add_contact(const Contact& contact) {
contacts_.push_back(contact);
}
// 显示所有联系人
void show_contacts() const {
for (const auto& contact : contacts_) {
cout << "姓名:" << contact.name() << ",电话:" << contact.phone() << ",地址:" << contact.address() << endl;
}
}
// 删除联系人
void remove_contact(const string& name) {
for (auto it = contacts_.begin(); it != contacts_.end(); ++it) {
if (it->name() == name) {
contacts_.erase(it);
break;
}
}
}
// 查找联系人
void find_contact(const string& name) const {
for (const auto& contact : contacts_) {
if (contact.name() == name) {
cout << "姓名:" << contact.name() << ",电话:" << contact.phone() << ",地址:" << contact.address() << endl;
return;
}
}
cout << "未找到联系人:" << name << endl;
}
// 修改联系人
void modify_contact(const string& name, const Contact& new_contact) {
for (auto& contact : contacts_) {
if (contact.name() == name) {
contact = new_contact;
return;
}
}
cout << "未找到联系人:" << name << endl;
}
// 清空联系人
void clear_contacts() {
contacts_.clear();
}
private:
vector<Contact> contacts_;
};
int main() {
ContactBook book;
while (true) {
// 显示菜单
cout << "请选择操作:" << endl;
cout << "1. 添加联系人" << endl;
cout << "2. 显示所有联系人" << endl;
cout << "3. 删除联系人" << endl;
cout << "4. 查找联系人" << endl;
cout << "5. 修改联系人" << endl;
cout << "6. 清空联系人" << endl;
cout << "7. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
string name, phone, address;
cout << "请输入姓名:";
cin >> name;
cout << "请输入电话:";
cin >> phone;
cout << "请输入地址:";
cin >> address;
book.add_contact(Contact(name, phone, address));
cout << "添加成功!" << endl;
break;
}
case 2:
book.show_contacts();
break;
case 3: {
string name;
cout << "请输入要删除的联系人姓名:";
cin >> name;
book.remove_contact(name);
break;
}
case 4: {
string name;
cout << "请输入要查找的联系人姓名:";
cin >> name;
book.find_contact(name);
break;
}
case 5: {
string name;
cout << "请输入要修改的联系人姓名:";
cin >> name;
string phone, address;
cout << "请输入新的电话:";
cin >> phone;
cout << "请输入新的地址:";
cin >> address;
book.modify_contact(name, Contact(name, phone, address));
break;
}
case 6:
book.clear_contacts();
cout << "清空成功!" << endl;
break;
case 7:
cout << "退出程序!" << endl;
return 0;
default:
cout << "无效的选择,请重新输入!" << endl;
break;
}
}
return 0;
}
```
以上代码可能并不是最优解,但能够满足你的需求。
相关推荐
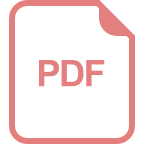
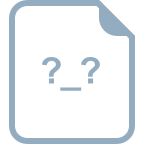
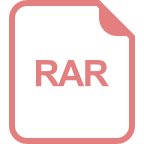









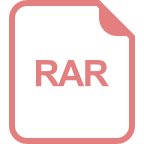
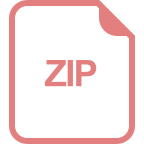
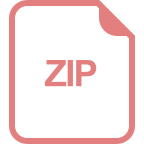
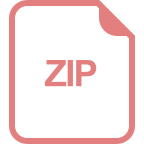