springboot上传文件保存到数据库
时间: 2023-08-25 10:27:17 浏览: 53
在Spring Boot中,可以使用Spring Data JPA和Hibernate实现将文件存储到数据库中。以下是实现文件上传并将其保存到数据库中的步骤:
1. 创建一个实体类,包含一个字段用于存储文件内容(一般使用byte[]类型),以及其他必要的字段。
```java
@Entity
public class FileEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String fileName;
private String fileType;
@Lob
private byte[] data;
// getters and setters
}
```
2. 创建一个控制器,用于处理文件上传请求。在控制器中,使用Spring的MultipartFile类来接收上传的文件,并将其保存到数据库中。
```java
@RestController
@RequestMapping("/api/files")
public class FileController {
@Autowired
private FileRepository fileRepository;
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
try {
// Create a new FileEntity object and set its fields
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(file.getOriginalFilename());
fileEntity.setFileType(file.getContentType());
fileEntity.setData(file.getBytes());
// Save the file to the database
fileRepository.save(fileEntity);
return new ResponseEntity<>("File uploaded successfully", HttpStatus.OK);
} catch (IOException e) {
e.printStackTrace();
return new ResponseEntity<>("Failed to upload file", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
3. 创建一个Spring Data JPA存储库,用于与数据库交互。在存储库中,定义一个方法用于保存FileEntity对象。
```java
@Repository
public interface FileRepository extends JpaRepository<FileEntity, Long> {
// Define any additional methods here
}
```
4. 在应用程序配置文件中,配置数据库连接信息和JPA属性。例如,如果使用MySQL数据库,可以添加以下属性:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=myusername
spring.datasource.password=mypassword
spring.jpa.hibernate.ddl-auto=update
```
这样,你就可以将上传的文件保存到数据库中了。当需要访问文件时,可以从数据库中检索FileEntity对象,并将其数据字段发送回客户端。
相关推荐
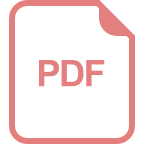














