c语言启动多个线程 ubuntu
时间: 2023-05-08 12:55:44 浏览: 124
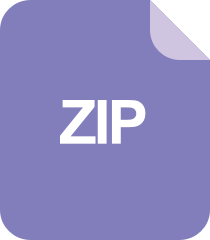
模拟FTP未删减.zip
在Ubuntu上启动多个C语言线程,可以使用pthread库。pthread库是C语言的多线程库,它可以为我们提供创建线程、线程同步、线程销毁等功能。
首先,在Ubuntu上使用终端打开你的C语言程序所在的目录,使用gcc编译器进行编译,例如:gcc –o thread thread.c –lpthread
然后,在C语言程序中引入头文件pthread.h,并定义所需的变量和结构体。由于pthread_create()函数用于创建线程,我们需要用它创建额外的线程。例如:pthread_t tid[2]; //线程标识符
接下来,在主线程中使用pthread_create()函数创建额外的线程,指定适当的参数和线程执行函数,例如:pthread_create(&tid[0], NULL, func1, NULL); pthread_create(&tid[1], NULL, func2, NULL);
其中,tid是线程标识符,func1和func2是线程执行函数,NULL是可选参数。
最后,在C语言程序中使用pthread_join()函数等待额外的线程完成,以防止主线程在子线程完成之前终止运行,例如:pthread_join(tid[0], NULL); pthread_join(tid[1], NULL);
以上就是在Ubuntu上启动多个C语言线程的基本步骤。同时,需要注意的是要正确使用互斥锁等同步机制,以避免线程访问共享资源时出现问题。
阅读全文
相关推荐
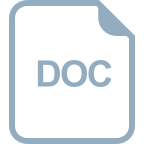
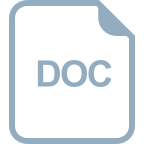


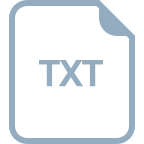
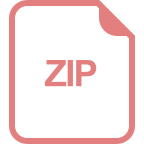
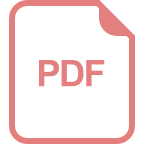
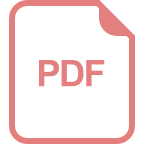
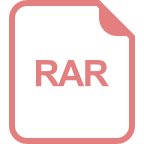
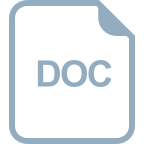
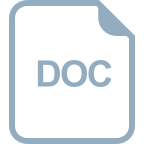
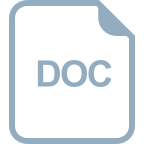
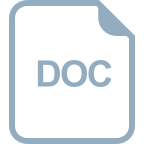
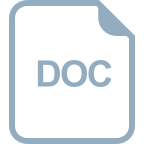
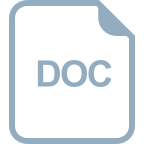
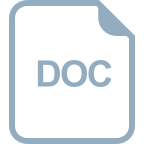
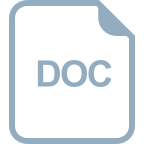
