(1)建立一个接口Ishape,包括了两个抽象方法,一个是求周长的方法length(),一个是求面积的方法area(); (2)新建一个实现类圆Circle继承Ishape (3)新建一个实现类矩形Rect继承Ishape (4)新建一个实现类三角形Triangle类继承Ishape (5)新建一个测试类Test,在里面通过接口回调实现多态
时间: 2023-12-03 18:43:40 浏览: 122
以下是实现Ishape接口和三个形状类的示例代码:
```java
// Ishape接口
public interface Ishape {
double length(); // 求周长的方法
double area(); // 求面积的方法
}
// 圆类
public class Circle implements Ishape {
private double radius; // 圆的半径
public Circle(double radius) {
this.radius = radius;
}
@Override
public double length() {
return 2 * Math.PI * radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
// 矩形类
public class Rect implements Ishape {
private double width; // 矩形的宽
private double height; // 矩形的高
public Rect(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double length() {
return 2 * (width + height);
}
@Override
public double area() {
return width * height;
}
}
// 三角形类
public class Triangle implements Ishape {
private double a; // 三角形的边长a
private double b; // 三角形的边长b
private double c; // 三角形的边长c
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
@Override
public double length() {
return a + b + c;
}
@Override
public double area() {
double p = (a + b + c) / 2; // 半周长
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
}
```
以下是使用多态实现的Test测试类示例代码:
```java
public class Test {
public static void main(String[] args) {
Ishape[] shapes = {new Circle(3), new Rect(4, 5), new Triangle(3, 4, 5)};
for (Ishape shape : shapes) {
System.out.println(shape.getClass().getSimpleName() + "周长为:" + shape.length());
System.out.println(shape.getClass().getSimpleName() + "面积为:" + shape.area());
}
}
}
```
输出结果为:
```
Circle周长为:18.84955592153876
Circle面积为:28.274333882308138
Rect周长为:18.0
Rect面积为:20.0
Triangle周长为:12.0
Triangle面积为:6.0
```
可以看到,通过接口回调实现多态,我们可以方便地对不同的形状进行周长和面积的计算。
阅读全文
相关推荐
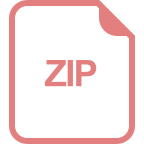
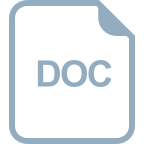
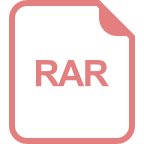
















