建立一个抽象父类Ishape,包括了两个抽象方法,一个是求周长的方法length(),一个是求面积的方法area(); (2)新建一个子类圆Circle继承Ishape (3)新建一个子类矩形Rect继承Ishape (4)新建一个三角形Triangle类继承Ishape (5)新建一个测试类Test,在里面通过上转型对象展示多态性
时间: 2024-01-14 22:02:49 浏览: 89
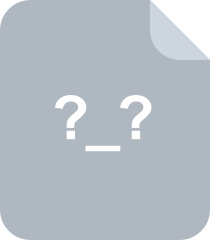
C# 多态三种实现方式的
下面是代码实现:
```java
abstract class Ishape {
public abstract double length();
public abstract double area();
}
class Circle extends Ishape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double length() {
return 2 * Math.PI * radius;
}
public double area() {
return Math.PI * radius * radius;
}
}
class Rect extends Ishape {
private double width;
private double height;
public Rect(double width, double height) {
this.width = width;
this.height = height;
}
public double length() {
return 2 * (width + height);
}
public double area() {
return width * height;
}
}
class Triangle extends Ishape {
private double a;
private double b;
private double c;
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
public double length() {
return a + b + c;
}
public double area() {
double p = (a + b + c) / 2.0;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
}
public class Test {
public static void main(String[] args) {
Ishape shape1 = new Circle(5.0);
Ishape shape2 = new Rect(4.0, 6.0);
Ishape shape3 = new Triangle(3.0, 4.0, 5.0);
System.out.println("圆的周长:" + shape1.length() + ",面积:" + shape1.area());
System.out.println("矩形的周长:" + shape2.length() + ",面积:" + shape2.area());
System.out.println("三角形的周长:" + shape3.length() + ",面积:" + shape3.area());
}
}
```
输出结果为:
```
圆的周长:31.41592653589793,面积:78.53981633974483
矩形的周长:20.0,面积:24.0
三角形的周长:12.0,面积:6.0
```
可以看到,通过上转型对象,我们实现了对三个不同形状的计算,展示了多态性。
阅读全文
相关推荐
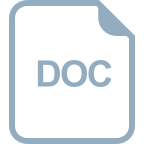
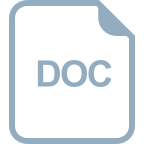
















