resttemplate发送https请求
时间: 2023-05-31 11:19:20 浏览: 124
### 回答1:
RestTemplate可以通过以下步骤发送HTTPS请求:
1. 创建SSL上下文
可以使用以下代码创建SSL上下文:
```
TrustStrategy acceptingTrustStrategy = (X509Certificate[] chain, String authType) -> true;
SSLContext sslContext = SSLContexts.custom()
.loadTrustMaterial(null, acceptingTrustStrategy)
.build();
```
这将创建一个信任所有证书的SSL上下文。
2. 创建HTTP客户端
可以使用以下代码创建HTTP客户端:
```
HttpClient client = HttpClients.custom()
.setSSLContext(sslContext)
.build();
```
这将创建一个使用SSL上下文的HTTP客户端。
3. 创建RestTemplate
可以使用以下代码创建RestTemplate:
```
RestTemplate restTemplate = new RestTemplate(new HttpComponentsClientHttpRequestFactory(client));
```
这将创建一个使用HTTP客户端的RestTemplate。
4. 发送HTTPS请求
可以使用以下代码发送HTTPS请求:
```
ResponseEntity<String> response = restTemplate.exchange(
"https://example.com",
HttpMethod.GET,
null,
String.class);
```
这将发送一个GET请求到https://example.com,并返回响应体的字符串表示。
### 回答2:
RestTemplate是一个Spring框架中的HTTP客户端,它支持发送HTTP和HTTPS请求。HTTPS是一种基于SSL加密通信协议的HTTP协议,它可以提供更高的网络安全性。RestTemplate发送HTTPS请求时需要进行一些配置。
首先,我们需要创建一个支持HTTPS的RestTemplate,并为其配置SSL/TLS证书。SSL/TLS证书用于验证服务器的身份,并确保通信数据的机密性。我们可以使用Java keytool工具生成证书,将其保存到keystore文件中,并将keystore文件加载到RestTemplate中:
```
KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType());
FileInputStream fileInputStream = new FileInputStream(new File("keystore.jks"));
keyStore.load(fileInputStream, "password".toCharArray());
SSLContext sslContext = SSLContextBuilder
.create()
.loadKeyMaterial(keyStore, "password".toCharArray())
.build();
CloseableHttpClient httpClient = HttpClients.custom()
.setSSLContext(sslContext)
.build();
HttpComponentsClientHttpRequestFactory requestFactory =
new HttpComponentsClientHttpRequestFactory();
requestFactory.setHttpClient(httpClient);
RestTemplate restTemplate = new RestTemplate(requestFactory);
```
这段代码中,我们创建了一个KeyStore对象并将其加载到RestTemplate中,然后使用SSLContextBuilder创建一个SSLContext对象,并使用KeyStore来加载证书。接着,我们使用HttpClients创建一个CloseableHttpClient对象,并将其设置为RestTemplate的请求工厂。最后,我们使用这个RestTemplate对象来发送HTTPS请求。
使用RestTemplate发送HTTPS请求时,我们还需要注意服务器证书的验证。在默认情况下,RestTemplate会执行服务器证书的验证,并抛出异常。我们可以通过配置RestTemplate来跳过服务器证书的验证:
```
TrustStrategy acceptingTrustStrategy = (X509Certificate[] chain, String authType) -> true;
SSLContext sslContext = SSLContextBuilder
.create()
.loadTrustMaterial(null, acceptingTrustStrategy)
.build();
CloseableHttpClient httpClient = HttpClients.custom()
.setSSLContext(sslContext)
.build();
HttpComponentsClientHttpRequestFactory requestFactory =
new HttpComponentsClientHttpRequestFactory();
requestFactory.setHttpClient(httpClient);
RestTemplate restTemplate = new RestTemplate(requestFactory);
```
这段代码中,我们创建了一个TrustStrategy对象来跳过服务器证书的验证。然后,我们使用SSLContextBuilder创建了一个SSLContext对象,并将其设置为RestTemplate的SSL上下文。最后,我们使用这个RestTemplate对象来发送HTTPS请求。
总之,使用RestTemplate发送HTTPS请求需要进行一些配置,包括SSL/TLS证书的配置和服务器证书的验证。我们可以通过配置RestTemplate来满足不同的安全需求。
### 回答3:
RestTemplate是一个基于HTTP协议的客户端请求工具,它可以用来发送HTTP请求。当需要发送HTTPS请求时,需要进行一些额外的配置,下面我们来了解一下如何使用RestTemplate发送HTTPS请求。
首先,我们需要导入证书到本地的证书库中,可以使用Java的keytool命令进行导入,执行以下命令:
keytool -import -alias mycert -file c:\mycert.cer -keystore c:\mykeystore.jks
其中,mycert是证书别名,mycert.cer是证书的路径,mykeystore.jks是证书库的路径。
然后,在代码中配置RestTemplate对HTTPS请求进行支持,可以通过创建一个SSL连接工厂,再将其设置到RestTemplate中的方式来实现,代码如下所示:
`private RestTemplate restTemplate() throws Exception {`
`TrustStrategy acceptingTrustStrategy = (X509Certificate[] chain, String authType) -> true;`
`SSLContext sslContext = SSLContextBuilder.create().loadTrustMaterial(acceptingTrustStrategy).build();`
`SSLConnectionSocketFactory csf = new SSLConnectionSocketFactory(sslContext);`
`CloseableHttpClient httpClient = HttpClients.custom().setSSLSocketFactory(csf).build();`
`HttpComponentsClientHttpRequestFactory requestFactory =`
`new HttpComponentsClientHttpRequestFactory();`
`requestFactory.setHttpClient(httpClient);`
`return new RestTemplate(requestFactory);`
`}`
在以上代码中,我们创建了一个TrustStrategy对象,它会信任所有证书。然后,我们使用该对象创建了一个SSLContext对象,利用该对象我们可以创建一个SSL连接工厂(即SSLConnectionSocketFactory)。接下来,我们使用该连接工厂创建了一个HttpClient对象,并将其设置为RestTemplate的请求工厂(即HttpComponentsClientHttpRequestFactory)。
最后,我们使用RestTemplate发送HTTPS请求,示例代码如下:
`public void requestHttps() throws Exception {`
`String url = "https://www.example.com/api";`
`ResponseEntity<String> responseEntity = restTemplate().exchange(url, HttpMethod.GET, null, String.class);`
`System.out.println(responseEntity.getBody());`
`}`
在示例代码中,我们使用exchange方法发送了一个GET请求,并将响应的数据打印到控制台上。
总之,使用RestTemplate发送HTTPS请求需要导入证书到本地证书库中,并对RestTemplate进行一些配置。RestTemplate提供了很多方法,我们可以根据具体的需求选择不同的方法发送不同类型的请求,更好地满足我们的开发需求。
阅读全文
相关推荐
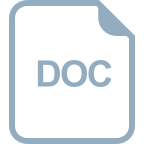
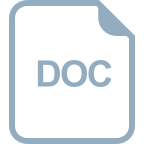
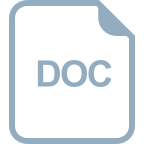















