[3].设计一个类UseCompute,含有方法: public void useCom(Compute com, int one, int two) 此方法要求能够:1.用传递过来的对象调用computer方法完成运算 2.输出运算的结果
时间: 2024-09-28 11:10:28 浏览: 58
在Java中,你可以设计一个名为`UseCompute`的类,这个类的作用是为了通用地利用一个实现了特定运算接口(如`Compute`)的对象来进行计算,并显示结果。假设`Compute`接口定义了一个`compute(int a, int b)`方法用于执行某种运算。下面是一个简单的实现:
```java
// 定义Compute接口
interface Compute {
int compute(int one, int two);
}
// 实现UseCompute类
class UseCompute {
// 使用Compute对象并调用compute方法
public void useCom(Compute com, int one, int two) {
int result = com.compute(one, two);
System.out.println("运算结果是: " + result);
}
}
```
在这个`useCom`方法中,我们接受一个`Compute`类型的参数`com`,然后通过`com.compute()`来调用具体的计算方法。无论`com`实际是什么类的实例,只要它遵守了`Compute`接口的规范,就能保证正确的运行。
相关问题
利用接口做参数,写个计算器,能完成加减乘除运算。 1.定义一个接口Compute含有一个方法int computer(int n, int m)。 2.设计四个类分别实现此接口,完成加减乘除运算。 3.设计一个类UseCompute,类中含有方法:public void useCom(Compute com, int one, int two),此方法能够用传递过来的对象调用computer方法完成运算,并输出运算的结果。 4.设计一个主类Test,调用UseCompute中的方法useCom来完成加减乘除运算。
好的,以下是代码实现:
1. 定义Compute接口
```java
public interface Compute {
int computer(int n, int m);
}
```
2. 实现加减乘除四个类
```java
public class Add implements Compute {
@Override
public int computer(int n, int m) {
return n + m;
}
}
public class Sub implements Compute {
@Override
public int computer(int n, int m) {
return n - m;
}
}
public class Mul implements Compute {
@Override
public int computer(int n, int m) {
return n * m;
}
}
public class Div implements Compute {
@Override
public int computer(int n, int m) {
if (m == 0) {
throw new IllegalArgumentException("除数不能为0");
}
return n / m;
}
}
```
3. 设计UseCompute类
```java
public class UseCompute {
public void useCom(Compute com, int one, int two) {
int result = com.computer(one, two);
System.out.println("运算结果为:" + result);
}
}
```
4. 设计Test主类
```java
public class Test {
public static void main(String[] args) {
UseCompute uc = new UseCompute();
int a = 10;
int b = 5;
Compute add = new Add();
Compute sub = new Sub();
Compute mul = new Mul();
Compute div = new Div();
uc.useCom(add, a, b);
uc.useCom(sub, a, b);
uc.useCom(mul, a, b);
uc.useCom(div, a, b);
}
}
```
这样就可以通过传递不同的Compute对象来完成不同的加减乘除运算了。
java利用接口做参数,写个计算器,能完成加减乘除运算。 (1)定义一个接口Compute含有一个方法int computer(int n, int m)。 (2)设计四个类分别实现此接口,完成加减乘除运算。 (3)设计一个类UseCompute,类中含有方法:public void useCom(Compute com, int one, int two),此方法能够用传递过来的对象调用computer方法完成运算,并输出运算的结果。 (4)设计一个主类Test,调用UseCompute中的方法useCom来完成加减乘除运算。
代码如下:
接口Compute:
```
public interface Compute {
int computer(int n, int m);
}
```
实现加法的类Add:
```
public class Add implements Compute {
public int computer(int n, int m) {
return n + m;
}
}
```
实现减法的类Sub:
```
public class Sub implements Compute {
public int computer(int n, int m) {
return n - m;
}
}
```
实现乘法的类Mul:
```
public class Mul implements Compute {
public int computer(int n, int m) {
return n * m;
}
}
```
实现除法的类Div:
```
public class Div implements Compute {
public int computer(int n, int m) {
if (m == 0) {
throw new IllegalArgumentException("除数不能为0");
}
return n / m;
}
}
```
使用接口Compute的类UseCompute:
```
public class UseCompute {
public void useCom(Compute com, int one, int two) {
int result = com.computer(one, two);
System.out.println("运算结果为:" + result);
}
}
```
主类Test:
```
public class Test {
public static void main(String[] args) {
UseCompute useCompute = new UseCompute();
Compute add = new Add();
Compute sub = new Sub();
Compute mul = new Mul();
Compute div = new Div();
useCompute.useCom(add, 10, 5);
useCompute.useCom(sub, 10, 5);
useCompute.useCom(mul, 10, 5);
useCompute.useCom(div, 10, 5);
}
}
```
输出结果为:
```
运算结果为:15
运算结果为:5
运算结果为:50
运算结果为:2
```
阅读全文
相关推荐
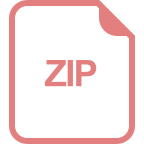
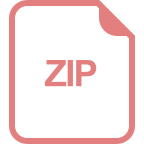
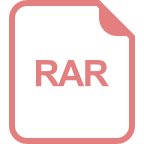

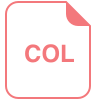
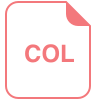
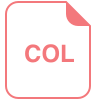









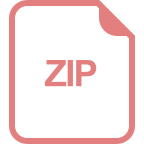