使用Python生成泰森多边形
时间: 2023-07-11 10:08:12 浏览: 337
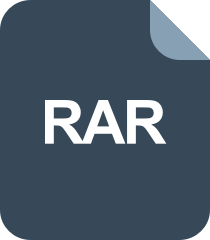
3D-voronoi_Voronoi3D_dirichlet图_python_泰森多边形_voronoi_

要生成泰森多边形,可以使用Python中的Delaunay三角剖分算法。下面是一个简单的示例代码:
```python
import numpy as np
from scipy.spatial import Delaunay
import matplotlib.pyplot as plt
# 生成随机点
points = np.random.rand(30, 2)
# 计算Delaunay三角剖分
tri = Delaunay(points)
# 绘制三角形
plt.triplot(points[:,0], points[:,1], tri.simplices)
plt.plot(points[:,0], points[:,1], 'o')
plt.show()
```
这个示例代码中,首先生成了30个随机点,然后使用`Delaunay`函数计算出它们的Delaunay三角剖分,最后使用`triplot`函数绘制出这些三角形。你可以根据需要修改点的数量和其他参数来生成不同形状的泰森多边形。
阅读全文
相关推荐
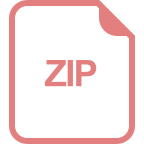
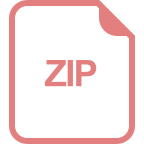









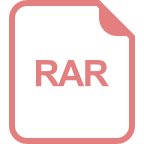
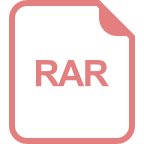
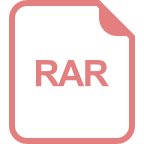
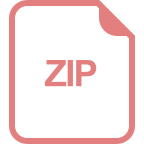
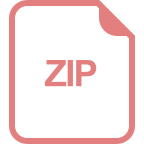
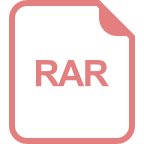