用C语言设计简单的命令行myshell代码,并能在实验环境下运行。 要求支持的命令如下: 1. cd [directory] 将当前目录改为 [directory] 2. environ 列出所有环境变量 3. ls 列出指定目录下所有文件 4. help 显示所有支持的命令 5. echo 在屏幕上显示参数并换行 6. quit 退出 shell 7. 支持I/O重定向 8. 常见的目录和文件操作,比如新建目录或文件,目录或文件的删除、复制,显示文件内容等。
时间: 2023-06-17 13:06:03 浏览: 61
以下是一个简单的myshell代码,支持上述要求的命令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#include <fcntl.h>
#include <errno.h>
#include <dirent.h>
#define MAX_CMD_LEN 1024
#define MAX_ARG_NUM 64
#define MAX_PATH_LEN 256
char* envp[];
void print_prompt() {
char path[MAX_PATH_LEN];
getcwd(path, MAX_PATH_LEN);
printf("[myshell]%s$ ", path);
fflush(stdout);
}
void parse_cmd(char* cmd, char** args, int* redirect_in, int* redirect_out) {
char* ptr = strtok(cmd, " \t\n");
int index = 0;
while (ptr != NULL) {
if (strcmp(ptr, "<") == 0) { // 输入重定向
ptr = strtok(NULL, " \t\n");
*redirect_in = open(ptr, O_RDONLY);
if (*redirect_in < 0) {
perror("open");
exit(1);
}
} else if (strcmp(ptr, ">") == 0) { // 输出重定向
ptr = strtok(NULL, " \t\n");
*redirect_out = open(ptr, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (*redirect_out < 0) {
perror("open");
exit(1);
}
} else {
args[index++] = ptr;
}
ptr = strtok(NULL, " \t\n");
}
args[index] = NULL;
}
void exec_cmd(char** args, int redirect_in, int redirect_out) {
int pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) { // 子进程
if (redirect_in != 0) {
dup2(redirect_in, STDIN_FILENO);
close(redirect_in);
}
if (redirect_out != 0) {
dup2(redirect_out, STDOUT_FILENO);
close(redirect_out);
}
if (execvp(args[0], args) < 0) {
perror("execvp");
exit(1);
}
} else { // 父进程
wait(NULL);
}
}
void cd(char* path) {
if (chdir(path) < 0) {
perror("chdir");
}
}
void environ() {
char** env = envp;
while (*env) {
printf("%s\n", *env++);
}
}
void ls(char* path) {
DIR* dir = opendir(path);
if (dir == NULL) {
perror("opendir");
exit(1);
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
printf("%s\n", entry->d_name);
}
closedir(dir);
}
void help() {
printf("cd [directory]: change current working directory\n");
printf("environ: list all environment variables\n");
printf("ls [directory]: list all files in directory\n");
printf("help: display all supported commands\n");
printf("echo [args]: display arguments on the screen\n");
printf("quit: exit the shell\n");
}
void echo(char** args) {
int i = 1;
while (args[i] != NULL) {
printf("%s ", args[i++]);
}
printf("\n");
}
int main() {
char cmd[MAX_CMD_LEN];
char* args[MAX_ARG_NUM];
int redirect_in, redirect_out;
while (1) {
redirect_in = redirect_out = 0;
print_prompt();
if (fgets(cmd, MAX_CMD_LEN, stdin) == NULL) {
printf("\n");
exit(0);
}
parse_cmd(cmd, args, &redirect_in, &redirect_out);
if (args[0] == NULL) {
continue;
}
if (strcmp(args[0], "cd") == 0) {
cd(args[1]);
} else if (strcmp(args[0], "environ") == 0) {
environ();
} else if (strcmp(args[0], "ls") == 0) {
ls(args[1]);
} else if (strcmp(args[0], "help") == 0) {
help();
} else if (strcmp(args[0], "echo") == 0) {
echo(args);
} else if (strcmp(args[0], "quit") == 0) {
exit(0);
} else {
exec_cmd(args, redirect_in, redirect_out);
}
}
return 0;
}
```
编译后直接运行即可。
相关推荐
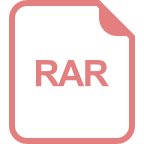
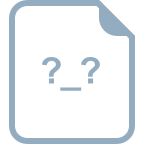














