``` people = {"last_name":"site","first_name":"li","age":"eighteen","city":"shanghai"} print = (people)```错误分析
时间: 2024-08-16 10:08:38 浏览: 30
在给出的代码片段中,存在一些语法错误和不当的操作。首先,`print = (people)` 的语法是错误的。在Python中,赋值运算符应该是 `=` 而不是圆括号 `()`,而且 `print` 是一个内置函数,不应该用于赋值。
正确的做法应该是打印这个字典,应该去掉圆括号,并使用花括号 `{}` 或者直接打印字典名:
```python
people = {"last_name": "site", "first_name": "li", "age": "eighteen", "city": "shanghai"}
# 正确的打印方式:
# 直接打印字典
print(people)
# 或者使用花括号作为字符串
print(f"people: {people}")
```
错误分析:
1. 括号 `()` 是不必要的,因为这里不需要调用 `print` 函数。
2. 应该将 `print` 命令修改为直接打印字典内容,或者通过字符串格式化输出。
3. 字典键值对的冒号后的值需要用双引号包围,而不是单引号。
相关问题
Write a program that asks the user for a two-digit number, then prints the English word for the number:
Sure, here's a possible solution in Python:
```
num = input("Please enter a two-digit number: ")
words = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen"]
tens = ["", "", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"]
if len(num) != 2:
print("Invalid input. Please enter a two-digit number.")
else:
if num[0] == "1":
print(words[int(num)])
else:
print(tens[int(num[0])] + "-" + words[int(num[1])])
```
This program first prompts the user to enter a two-digit number. It then checks if the input is valid (i.e., has exactly two digits). If the input is valid, it converts the number to its English word representation. For example, if the user enters "42", the program outputs "forty-two". If the user enters "13", the program outputs "thirteen".
编写一个程序,要求用户输入一个两位数,然后输出显示该数的英文单词。提示:把数分解为两个数字。用一个switch语句显示第一位数字对应的单词(“twenty”、“thirty”等),用第二个switch语句显示第二位数字对应的单词。不要忘记11~19需要特殊处理。
好的,以下是编写一个程序,要求用户输入一个两位数,然后输出显示该数的英文单词的代码:
```python
num = int(input("请输入一个两位数:"))
# 分解数字
tens = num // 10
ones = num % 10
# 处理特殊情况
if tens == 1:
if ones == :
print("ten")
elif ones == 1:
print("eleven")
elif ones == 2:
print("twelve")
elif ones == 3:
print("thirteen")
elif ones == 4:
print("fourteen")
elif ones == 5:
print("fifteen")
elif ones == 6:
print("sixteen")
elif ones == 7:
print("seventeen")
elif ones == 8:
print("eighteen")
elif ones == 9:
print("nineteen")
else:
# 处理十位数字
if tens == 2:
print("twenty", end=" ")
elif tens == 3:
print("thirty", end=" ")
elif tens == 4:
print("forty", end=" ")
elif tens == 5:
print("fifty", end=" ")
elif tens == 6:
print("sixty", end=" ")
elif tens == 7:
print("seventy", end=" ")
elif tens == 8:
print("eighty", end=" ")
elif tens == 9:
print("ninety", end=" ")
# 处理个位数字
if ones == 1:
print("one")
elif ones == 2:
print("two")
elif ones == 3:
print("three")
elif ones == 4:
print("four")
elif ones == 5:
print("five")
elif ones == 6:
print("six")
elif ones == 7:
print("seven")
elif ones == 8:
print("eight")
elif ones == 9:
print("nine")
```
希望能帮到您!
相关推荐
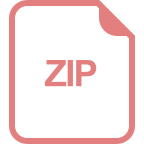
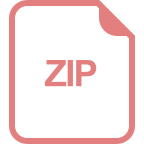
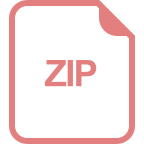












