做一个自己的时钟页面(可用HTML5+CSS3 或者Web UI库/框架),可创新设计时钟界面
时间: 2024-09-29 10:12:59 浏览: 102
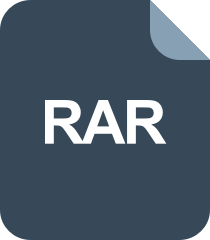
js+css3智能手表时钟代码
创建一个个性化的时钟页面,你可以结合HTML5、CSS3和JavaScript(或者现代前端UI库如Bootstrap、Materialize CSS等),按照以下步骤进行:
1. **HTML结构**:
- 使用`<div>`作为容器,可以包含小时、分钟和秒的部分。
- 可以使用`<span>`标签表示各个数字,并添加伪元素(`:before`和`:after`)来制作指针效果。
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="clock-container">
<div id="hour-hand"></div>
<div id="minute-hand"></div>
<div id="second-hand"></div>
<div class="time-text">00:00:00</div>
</div>
<script src="script.js"></script>
</body>
</html>
```
2. **CSS样式 (styles.css)**:
- 设计时钟背景、字体颜色以及指针样式。
- 利用CSS动画让指针旋转。
```css
.clock-container {
display: flex;
justify-content: center;
align-items: center;
height: 300px;
width: 300px;
border-radius: 50%;
background-color: #f8f9fa;
}
.time-text {
font-size: 48px;
color: white;
text-align: center;
}
.hand {
position: absolute;
transform-origin: bottom;
}
```
3. **JavaScript (script.js) - 动画部分**:
- 定义一个函数定时更新时间并改变指针位置。
- 使用`requestAnimationFrame`确保平滑的动画效果。
```javascript
const hourHand = document.getElementById('hour-hand');
const minuteHand = document.getElementById('minute-hand');
const secondHand = document.getElementById('second-hand');
function updateClock() {
const now = new Date();
const hours = now.getHours();
const minutes = now.getMinutes();
const seconds = now.getSeconds();
// 将小时转换为角度
let hourAngle = ((hours % 12) + minutes / 60 + seconds / 3600) * 360;
// 更新指针位置
hourHand.style.transform = `rotate(${hourAngle}deg)`;
minuteHand.style.transform = `rotate(${minutes * 6}deg)`;
secondHand.style.transform = `rotate(${seconds * 360}deg)`;
// 每秒钟更新一次
requestAnimationFrame(updateClock);
}
updateClock();
```
4. **创新设计**:
- 可以尝试改变颜色主题、动态背景、圆形或非圆形布局、数字显示风格(比如渐变色或立体效果)、添加日期信息或自定义图标等等。
相关问题:
1. 如何使用CSS3做更复杂的时钟动画?
2. 怎么给这个时钟添加交互性,例如点击切换主题?
3. HTML5有哪些新特性可用于改进这个时钟项目?
阅读全文
相关推荐
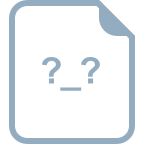
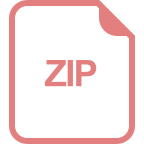
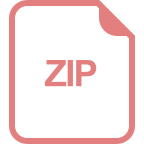
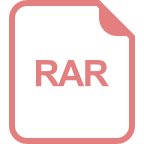
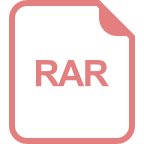
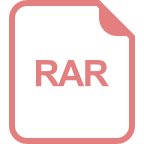
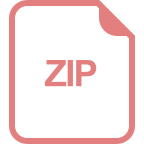
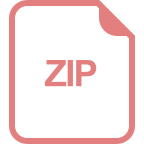
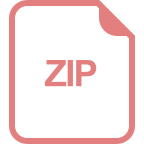
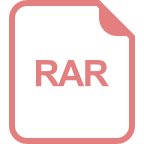
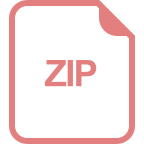
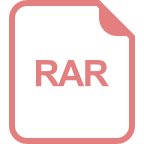
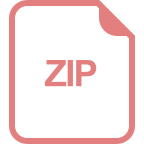
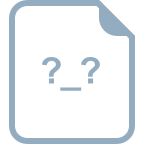
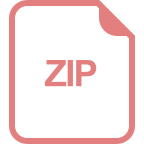
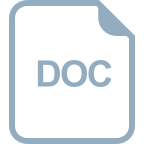
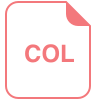
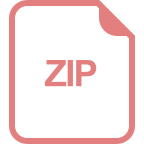