如何实现A*算法迷宫问题
时间: 2023-12-04 09:38:06 浏览: 42
A*算法是一种常用的路径搜索算法,可以用于解决迷宫问题。下面是使用C语言实现A*算法解决迷宫问题的基本步骤:
1. 定义迷宫地图:可以使用二维数组来表示迷宫地图,其中0表示可以通过的路,1表示障碍物。
2. 定义节点结构体:节点包含当前位置、父节点、G值、H值和F值等信息。
3. 定义open表和close表:open表用于存储待扩展的节点,close表用于存储已经扩展过的节点。
4. 初始化起点和终点:将起点加入open表中,并将其G值、H值和F值初始化为0。
5. 进入循环:每次从open表中取出F值最小的节点进行扩展,将其加入close表中,并将其周围的节点加入open表中。
6. 判断终点是否在close表中:如果终点在close表中,则搜索结束,否则返回步骤5。
7. 回溯路径:从终点开始,沿着每个节点的父节点一直回溯到起点,即可得到最短路径。
下面是一个简单的C语言实现A*算法解决迷宫问题的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ROW 10
#define COL 10
int map[ROW][COL] = {
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 1, 1, 1, 0, 1, 0},
{0, 1, 1, 0, 1, 1, 1, 0, 1, 0},
{0, 1, 1, 1, 1, 0, 0, 1, 1, 0},
{0, 1, 0, 1, 0, 1, 1, 1, 1, 0},
{0, 1, 1, 1, 0, 1, 1, 1, 1, 0},
{0, 1, 0, 1, 1, 1, 0, 1, 1, 0},
{0, 1, 0, 0, 0, 1, 1, 1, 1, 0},
{0, 1, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
};
typedef struct node {
int x;
int y;
struct node *parent;
int G;
int H;
int F;
} Node;
Node *openList[ROW * COL];
Node *closeList[ROW * COL];
int openCount = 0;
int closeCount = 0;
int getH(int x, int y, int endX, int endY) {
return abs(endX - x) + abs(endY - y);
}
bool isInCloseList(int x, int y) {
for (int i = 0; i < closeCount; i++) {
if (closeList[i]->x == x && closeList[i]->y == y) {
return true;
}
}
return false;
}
bool isInOpenList(int x, int y) {
for (int i = 0; i < openCount; i++) {
if (openList[i]->x == x && openList[i]->y == y) {
return true; }
}
return false;
}
void addNodeToOpenList(Node *node) {
openList[openCount++] = node;
}
void removeNodeFromOpenList(Node *node) {
for (int i = 0; i < openCount; i++) {
if (openList[i] == node) {
openList[i] = openList[--openCount];
break;
}
}
}
void addNodeToCloseList(Node *node) {
closeList[closeCount++] = node;
}
Node *getMinFNodeFromOpenList() {
Node *minNode = openList[0];
for (int i = 1; i < openCount; i++) {
if (openList[i]->F < minNode->F) {
minNode = openList[i];
}
}
return minNode;
}
void printPath(Node *node) {
if (node->parent != NULL) {
printPath(node->parent);
}
printf("(%d, %d) ", node->x, node->y);
}
void findPath(int startX, int startY, int endX, int endY) {
Node *startNode = (Node *)malloc(sizeof(Node));
startNode->x = startX;
startNode->y = startY;
startNode->parent = NULL;
startNode->G = 0;
startNode->H = getH(startX, startY, endX, endY);
startNode->F = startNode->G + startNode->H;
addNodeToOpenList(startNode);
while (openCount > 0) {
Node *currentNode = getMinFNodeFromOpenList();
removeNodeFromOpenList(currentNode);
addNodeToCloseList(currentNode);
if (currentNode->x == endX && currentNode->y == endY) {
printf("Path found: ");
printPath(currentNode);
printf("\n");
return;
}
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (i == 0 && j == 0) {
continue;
}
int x = currentNode->x + i;
int y = currentNode->y + j;
if (x < 0 || x >= ROW || y < 0 || y >= COL) {
continue;
}
if (map[x][y] == 1 || isInCloseList(x, y)) {
continue;
}
int G = currentNode->G + 1;
int H = getH(x, y, endX, endY);
int F = G + H;
if (isInOpenList(x, y)) {
for (int k = 0; k < openCount; k++) {
if (openList[k]->x == x && openList[k]->y == y) {
if (F < openList[k]->F) {
openList[k]->parent = currentNode;
openList[k]->G = G;
openList[k]->H = H;
openList[k]->F = F;
}
break;
}
}
} else {
Node *node = (Node *)malloc(sizeof(Node));
node->x = x;
node->y = y;
node->parent = currentNode;
node->G = G;
node->H = H;
node->F = F;
addNodeToOpenList(node);
}
}
}
}
printf("Path not found.\n");
}
int main() {
findPath(1, 1, 8, 8);
return 0;
}
```
相关推荐
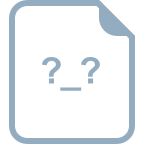
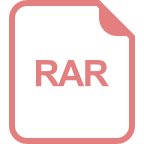













