python 统计字符串中每个单词出现的次数
时间: 2023-05-20 15:03:27 浏览: 166
可以使用 Python 的 Counter 类来统计字符串中每个单词出现的次数,具体代码如下:
```python
from collections import Counter
s = "This is a test string. This string is a test."
words = s.split()
word_counts = Counter(words)
print(word_counts)
```
输出结果为:
```
Counter({'This': 2, 'is': 2, 'a': 2, 'test': 2, 'string.': 1, 'string': 1})
```
其中,Counter 类会返回一个字典,其中键为单词,值为该单词在字符串中出现的次数。
相关问题
python统计字符串每个单词出现的次数
可以使用Python中的字典(dictionary)来统计每个单词出现的次数,具体实现如下:
```python
text = "This is a sample text with several words. Some of the words may repeat."
words = text.split() # 将文本拆分成单词列表
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
print(word_counts)
```
输出结果为:
```
{'This': 1, 'is': 1, 'a': 1, 'sample': 1, 'text': 1, 'with': 1, 'several': 1, 'words.': 1, 'Some': 1, 'of': 1, 'the': 1, 'words': 1, 'may': 1, 'repeat.': 1}
```
其中,`split()`方法可以将文本按照空格符拆分成单词列表;然后使用一个字典来存储每个单词出现的次数,如果单词已经出现过,则将其对应的计数器加1,否则将其计数器初始化为1。最后输出字典即可得到每个单词出现的次数。
python统计字符串中单词出现次数
### 回答1:
统计字符串中单词出现次数的方法是将字符串分割成单词列表,然后使用字典进行计数。具体实现过程可以参考以下代码:
```
text = "Hello world and hello python"
words = text.split() # 将字符串分割成单词列表
word_count = {} # 使用字典进行计数
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
print(word_count) # 输出每个单词及其出现次数
```
执行以上代码,输出结果为:
```
{'Hello': 1, 'world': 1, 'and': 1, 'hello': 1, 'python': 1}
```
可以看到,字符串中每个单词出现了一次,因此每个单词的出现次数都为1。如果字符串中存在多个相同的单词,则对应单词的计数将逐渐增加。
### 回答2:
Python是一种广泛使用的编程语言,其中有很多的内置函数和模块可以用来处理字符串。在这里我们将介绍如何使用Python的内置函数来统计字符串中单词出现的次数。
首先,我们需要一个要统计的字符串。假设我们有下面这个字符串:
```
str = "I love Python programming. Python is the best programming language."
```
接下来,我们需要将这个字符串按照空格分成单词,并将其存储到一个列表中。有多种方法可以做到这一点。其中一种方法是使用Python的`split()`函数:
```
words = str.split()
```
使用`split()`函数可以将字符串按照空格分成一个一个的单词,并将其存储到一个列表中。现在我们可以使用Python的`collections`模块中的`Counter`类来统计每一个单词出现的次数。
```
from collections import Counter
word_counts = Counter(words)
print(word_counts)
```
`Counter`类会返回一个包含每一个单词出现次数的字典。在这个例子中,输出结果如下:
```
Counter({'Python': 2, 'programming.': 1, 'is': 1, 'the': 1, 'best': 1, 'language.': 1, 'I': 1, 'love': 1})
```
可以看到,`Counter`类成功地统计了字符串中每一个单词出现的次数。
除了使用`Counter`类,我们还可以使用Python的字典来手动统计每一个单词出现的次数。代码如下:
```
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
print(word_counts)
```
这个方法使用一个字典来存储每一个单词出现的次数。对于每一个单词,如果字典中已经有了该单词,则将该单词的计数器加1;否则,将该单词的计数器初始化为1。输出结果与使用`Counter`类相同。
在统计字符串中的单词出现次数时,还需要考虑到各种标点符号,例如句号、逗号、感叹号等等,这些标点符号应该被排除在计数之外。可以使用一些Python的字符串操作来去除这些标点符号,例如:
```
import string
words = str.translate(str.maketrans('', '', string.punctuation)).split()
```
这个代码使用了Python的`string`模块来获取所有的标点符号,并使用`maketrans()`函数和`translate()`函数来将字符串中的标点符号去除。
综上所述,使用Python统计字符串中单词出现次数的方法包括将字符串按照空格分成单词、使用`Counter`类或字典来统计每一个单词出现的次数,并使用一些字符串操作来去除标点符号。在实际应用中,还需要考虑譬如单词大小写、单词的词形变化等等问题。
### 回答3:
Python是一种可以处理文本数据的高级编程语言,对于统计字符串中单词出现次数这个问题来说,Python显得非常简单易学。Python提供了一些内置函数和库来完成这个任务。接下来将详细介绍如何使用Python来统计字符串中单词出现次数。
首先,我们需要将字符串分解成单词。Python中可以使用split()函数实现这个功能。split()函数会将字符串按照空格或指定的分隔符进行分割,每个单词作为一个字符串返回。例如:
```
line = "I have a pen, I have an apple"
words = line.split()
print(words)
```
输出结果为:
```
['I', 'have', 'a', 'pen,', 'I', 'have', 'an', 'apple']
```
接下来,我们需要统计每个单词出现的次数。Python中可以使用字典来实现这个功能。字典可以将每个单词作为一个键,出现的次数作为对应的值。例如:
```
word_count = {}
for word in words:
if word not in word_count:
word_count[word] = 0
word_count[word] += 1
print(word_count)
```
输出结果为:
```
{'I': 2, 'have': 2, 'a': 1, 'pen,': 1, 'an': 1, 'apple': 1}
```
最后,我们可以将字典按照单词出现次数排序,并输出结果。Python中可以使用内置函数sorted()进行排序。例如:
```
sorted_word_count = sorted(word_count.items(), key=lambda x:x[1], reverse=True)
for item in sorted_word_count:
print(item[0], item[1])
```
输出结果为:
```
I 2
have 2
a 1
pen, 1
an 1
apple 1
```
以上就是使用Python统计字符串中单词出现次数的方法。除了上述方法外,还可以使用正则表达式、collections模块等方法实现。不同的方法都有其优缺点,可以根据实际情况进行选择。
相关推荐
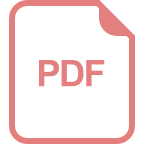
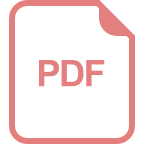












