python统计字符串中每个单词(不区分大小写)出现的次数。
时间: 2023-11-15 12:04:30 浏览: 68
可以使用 Python 内置的 Counter 类来实现:
```python
from collections import Counter
def count_words(text):
# 将所有单词转换为小写并用空格分隔
words = text.lower().split()
# 使用 Counter 统计单词出现次数
word_counts = Counter(words)
return word_counts
```
使用示例:
```python
text = "Python is an interpreted, high-level, general-purpose programming language."
word_counts = count_words(text)
print(word_counts)
```
输出结果:
```
Counter({'python': 1, 'is': 1, 'an': 1, 'interpreted,': 1, 'high-level,': 1, 'general-purpose': 1, 'programming': 1, 'language.': 1})
```
其中,Counter 对象的 key 为单词,value 为单词出现的次数。
相关问题
用python编写程序:统计输入的字符串中每个英文单词出现的次数,并以字典形式输出。单词中的字母不区分大小写。
可以使用 Python 的字典来实现统计每个英文单词出现次数的功能。具体的步骤如下:
1. 读取输入的字符串。
2. 将字符串转换为小写,以便统计单词时不区分大小写。
3. 使用正则表达式(re 模块)将字符串拆分为单词列表。
4. 遍历单词列表,统计每个单词出现的次数,并将结果存储在字典中。
5. 输出字典。
下面是代码示例:
```python
import re
# 读取输入的字符串
input_str = input("请输入字符串:")
# 将字符串转换为小写
input_str = input_str.lower()
# 使用正则表达式拆分字符串为单词列表
word_list = re.findall(r'\b\w+\b', input_str)
# 统计每个单词出现的次数
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 输出结果
print("每个单词出现的次数为:")
print(word_count)
```
注意,上述代码使用了正则表达式 `\b\w+\b` 来匹配字符串中的单词。`\b` 表示单词的边界,`\w+` 表示一个或多个字母数字字符。
统计输入的字符串中每个英文单词出现的次数,并以列表形式输出。单词中的字母不区分大小写。
好的,我可以帮你实现这个功能。以下是Python代码实现:
```python
def count_words(s):
word_dict = {}
words = s.lower().split()
for word in words:
if word.isalpha():
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict.items()
s = input("请输入字符串:")
result = count_words(s)
print(result)
```
代码实现的思路是先将输入的字符串转换为小写,并以空格为分隔符分割出单词。然后遍历每个单词,如果该单词只包含字母,则将其添加到字典中,并记录出现次数。最后返回字典中的项列表,即每个单词和出现次数的键值对。
相关推荐
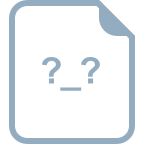













