用c写一个可执行递归下降分析程序
时间: 2024-04-30 11:20:08 浏览: 58
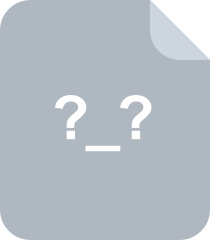
递归下降分析程序
下面是一个简单的可执行的递归下降分析程序,它可以解析一个简单的四则运算表达式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
char lookahead;
void error(char *msg) {
fprintf(stderr, "Error: %s\n", msg);
exit(1);
}
void match(char c) {
if (lookahead == c) {
lookahead = getchar();
} else {
char msg[32];
sprintf(msg, "Expected '%c'", c);
error(msg);
}
}
int factor() {
int result;
if (isdigit(lookahead)) {
result = lookahead - '0';
match(lookahead);
} else if (lookahead == '(') {
match('(');
result = expr();
match(')');
} else {
error("Expected digit or '('");
}
return result;
}
int term() {
int result = factor();
while (lookahead == '*' || lookahead == '/') {
if (lookahead == '*') {
match('*');
result *= factor();
} else {
match('/');
result /= factor();
}
}
return result;
}
int expr() {
int result = term();
while (lookahead == '+' || lookahead == '-') {
if (lookahead == '+') {
match('+');
result += term();
} else {
match('-');
result -= term();
}
}
return result;
}
int main() {
lookahead = getchar();
int result = expr();
if (lookahead != '\n') {
error("Unexpected input");
}
printf("Result: %d\n", result);
return 0;
}
```
这个程序中,我们使用 `lookahead` 变量来保存当前读入的字符。在 `match` 函数中,我们检查 `lookahead` 是否等于期望的字符,如果不是就报错。在 `factor` 函数中,我们首先检查 `lookahead` 是否为数字或左括号,如果是数字,就返回该数字;否则,就递归调用 `expr` 函数来计算括号内的表达式。在 `term` 函数中,我们首先计算一个因子,然后循环处理可能存在的乘法或除法操作。在 `expr` 函数中,我们首先计算一个项,然后循环处理可能存在的加法或减法操作。
在 `main` 函数中,我们首先读入一个字符作为 `lookahead`,然后调用 `expr` 函数来计算整个表达式的值。最后,我们检查 `lookahead` 是否为换行符,如果不是就报错。
阅读全文
相关推荐
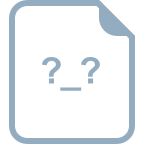
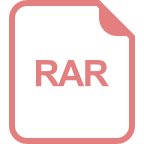
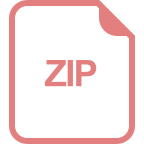
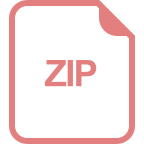
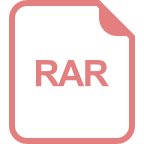
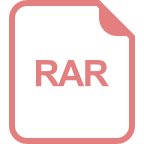
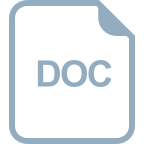
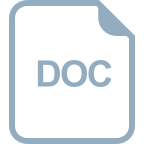
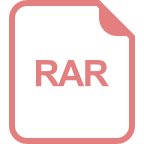
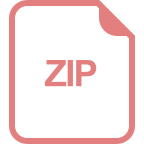
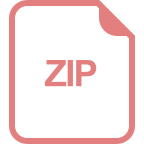
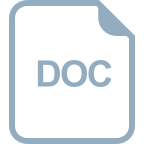
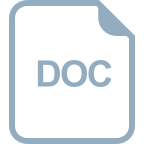
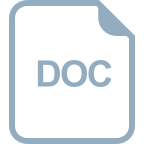
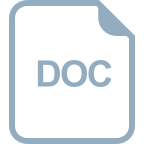
