Python 向西门子PLC发送
时间: 2023-06-24 10:09:14 浏览: 203
要在Python中向西门子PLC发送数据,可以使用S7comm协议和Python S7comm库。以下是一个发送数据到PLC的示例代码:
```python
from snap7.client import Client
# 连接PLC
plc = Client()
plc.connect('192.168.1.10', 0, 1)
# 发送数据
data = bytearray([1, 2, 3, 4])
plc.write_area(0x82, 0, 10, data)
# 断开连接
plc.disconnect()
```
在这个例子中,我们使用Python S7comm库中的`Client`类来连接PLC。我们指定PLC的IP地址和端口号,并调用`connect()`方法来建立连接。
然后,我们创建一个`bytearray`对象来存储要发送的数据,并使用`write_area()`方法将数据写入PLC的内存区域。
最后,我们使用`disconnect()`方法关闭连接。
请注意,PLC必须已经配置好S7comm通信,并且您需要知道要写入的内存区域的地址。
相关问题
python 向西门子PLC写入字符串
你可以使用 Python 的 Snap7 库来向西门子 PLC 写入字符串。以下是一个示例代码:
```python
import snap7
# 连接到 PLC
plc = snap7.client.Client()
plc.connect('192.168.1.1', 0, 1)
# 写入字符串
plc.db_write(1, 0, 'Hello, PLC!')
# 断开连接
plc.disconnect()
```
在上面的代码中,我们使用 `snap7.client.Client()` 函数连接到 PLC,并使用 `plc.db_write(1, 0, 'Hello, PLC!')` 函数向 DB1 的第0个字节写入字符串 "Hello, PLC!"。最后,我们使用 `plc.disconnect()` 函数断开连接。需要注意的是,你需要将代码中的 IP 地址 `192.168.1.1` 替换为你的 PLC 的 IP 地址。
python与西门子plc通信
Python与西门子PLC(Programmable Logic Controller)通信通常通过第三方库来实现,因为西门子PLC有自己的专用通信协议如MPI、PROFIBUS、MODBUS等。其中最常用的是S7-PLC库,比如`pycomm`(也叫`pypi.siemens.com`),它支持多种通信方式,包括TCP/IP、MPI和USB。
以下是一个简单的步骤概述:
1. 安装必要的库:首先需要安装`pycomm`库,可以使用pip命令进行安装:
```
pip install pycomm
```
2. 连接到PLC:创建`S7Connection`实例,并指定相应的设备地址和通信参数,例如:
```python
from pycomm import S7Connection
conn = S7Connection('192.168.1.100', 1) # 根据实际情况修改IP和端口
conn.connect()
```
3. 发送请求:根据PLC的数据结构(如输入/输出点、数据块等)发送读取或设置指令:
```python
data_input = conn.read_area('I', 1, 10) # 读取10个输入点
data_output = conn.write_area('Q', 1, [1, 0, 1]) # 设置前两个输出点
```
4. 关闭连接:操作完成后记得关闭连接:
```python
conn.disconnect()
```
阅读全文
相关推荐
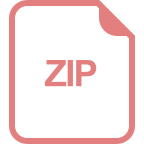
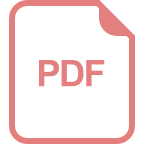
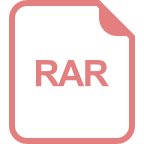













