In preparation for a class Fraction, create a class NullDenominatorException that must be a checked exception whose instances are thrown if the denominator of an object of class Fraction is 0.
时间: 2024-03-08 16:45:48 浏览: 24
Sure, here's an example implementation of the NullDenominatorException class in Java:
```
public class NullDenominatorException extends Exception {
public NullDenominatorException() {
super("Denominator cannot be null or zero.");
}
}
```
In this implementation, we extend the built-in Exception class to create our custom exception. We also provide a constructor that sets the exception message to a default value of "Denominator cannot be null or zero.". This message will be displayed when the exception is thrown.
To use this exception in our Fraction class, we can add a check for a null or zero denominator in the constructor and throw the exception if necessary. Here's an example implementation of the Fraction class:
```
public class Fraction {
private int numerator;
private int denominator;
public Fraction(int numerator, int denominator) throws NullDenominatorException {
if (denominator == 0) {
throw new NullDenominatorException();
}
this.numerator = numerator;
this.denominator = denominator;
}
// other methods for adding, subtracting, multiplying, and dividing Fractions
}
```
In this implementation, we check if the denominator is 0 and throw a NullDenominatorException if it is. Otherwise, we set the numerator and denominator instance variables and continue with the construction of the Fraction object.
相关推荐
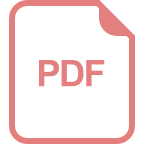
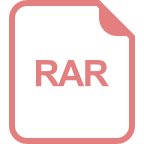
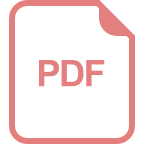














