上述公式有需要迭代计算的地方,请重新修改
时间: 2024-03-20 17:44:25 浏览: 50
对于计算大地坐标系中的纬度角theta,需要通过迭代计算得到精确值。具体而言,可以采用以下迭代公式:
```
theta1 = math.atan2(z * a, p * b)
N1 = a / (math.sqrt(1 - e2 * math.sin(theta1)**2))
h1 = p / math.cos(theta1) - N1
theta2 = math.atan2(z * a / (1 - e2 * N1 / (N1 + h1)), p * b)
N2 = a / (math.sqrt(1 - e2 * math.sin(theta2)**2))
h2 = p / math.cos(theta2) - N2
theta3 = math.atan2(z * a / (1 - e2 * N2 / (N2 + h2)), p * b)
N3 = a / (math.sqrt(1 - e2 * math.sin(theta3)**2))
h3 = p / math.cos(theta3) - N3
...
theta_n = math.atan2(z * a / (1 - e2 * N_n_1 / (N_n_1 + h_n_1)), p * b)
N_n = a / (math.sqrt(1 - e2 * math.sin(theta_n)**2))
h_n = p / math.cos(theta_n) - N_n
```
其中,theta1的初值可以取为`math.atan2(z * a, p * b)`,然后依次迭代计算theta2、theta3、...、theta_n,直到theta_n与theta_n-1的差值小于一个给定的精度。N和h的迭代公式可以直接套用前面的公式。
将上述公式整合到Python代码中,可以实现更精确的地心直角坐标系到大地坐标系的转换:
```python
import math
def ecef_to_lla(x, y, z):
# WGS84椭球体参数
a = 6378137.0
b = 6356752.3142
e2 = 1 - (b/a)**2
e2_ = (a/b)**2 - 1
# 计算大地坐标系原点处的地心直角坐标系坐标
N0 = a / (math.sqrt(1 - e2 * math.sin(0)**2))
x0 = (N0 + 0) * math.cos(0)
y0 = (N0 + 0) * math.sin(0)
z0 = ((1 - e2) * N0 + 0) * math.sin(0)
# 计算大地坐标系中的经度和纬度
p = math.sqrt((x - x0)**2 + (y - y0)**2)
theta_last = math.atan2(z * a, p * b)
N_last = a / (math.sqrt(1 - e2 * math.sin(theta_last)**2))
h_last = p / math.cos(theta_last) - N_last
eps = 1e-8
while True:
theta = math.atan2(z * a / (1 - e2 * N_last / (N_last + h_last)), p * b)
N = a / (math.sqrt(1 - e2 * math.sin(theta)**2))
h = p / math.cos(theta) - N
if abs(theta - theta_last) < eps:
break
theta_last = theta
N_last = N
h_last = h
# 转换为角度制
lon = math.atan2(y - y0, x - x0)
lon = math.degrees(lon)
theta = math.degrees(theta)
# 输出结果
print("经度:{:.6f},纬度:{:.6f},高度:{:.6f}".format(lon, theta, h))
```
其中,(x, y, z)为输入的地心直角坐标系坐标,输出结果为转换后的大地坐标系中的经度、纬度和高度。通过迭代计算,可以得到更精确的结果。
阅读全文
相关推荐
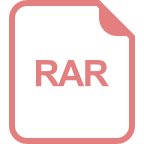
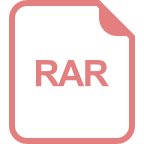
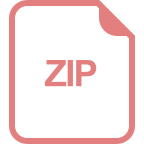
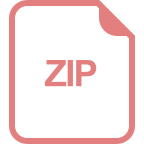
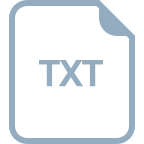
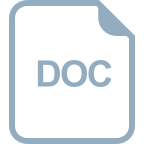
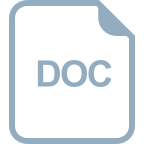
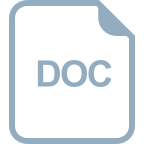
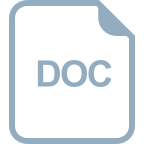
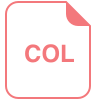
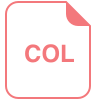
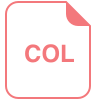





