写个多线程下载https资源的java代码
时间: 2023-03-23 18:04:13 浏览: 55
好的,以下是多线程下载https资源的Java代码:
```
import java.io.*;
import java.net.*;
import java.util.concurrent.*;
public class MultiThreadedDownloader {
private static final int BUFFER_SIZE = 4096;
private static final int MAX_THREADS = 10;
public static void download(String urlStr, String savePath) throws IOException {
URL url = new URL(urlStr);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
int contentLength = conn.getContentLength();
int numThreads = Math.min(MAX_THREADS, contentLength / BUFFER_SIZE);
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
int startPos = ;
int partSize = contentLength / numThreads;
for (int i = ; i < numThreads; i++) {
int endPos = startPos + partSize - 1;
if (i == numThreads - 1) {
endPos = contentLength - 1;
}
executor.execute(new DownloadThread(url, startPos, endPos, savePath));
startPos += partSize;
}
executor.shutdown();
try {
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS);
} catch (InterruptedException e) {
e.printStackTrace();
}
} else {
throw new IOException("Server returned HTTP response code: " + responseCode);
}
}
private static class DownloadThread implements Runnable {
private URL url;
private int startPos;
private int endPos;
private String savePath;
public DownloadThread(URL url, int startPos, int endPos, String savePath) {
this.url = url;
this.startPos = startPos;
this.endPos = endPos;
this.savePath = savePath;
}
@Override
public void run() {
try {
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Range", "bytes=" + startPos + "-" + endPos);
InputStream inputStream = conn.getInputStream();
RandomAccessFile file = new RandomAccessFile(savePath, "rw");
file.seek(startPos);
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
file.write(buffer, , bytesRead);
}
file.close();
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
希望能对您有所帮助!
相关推荐
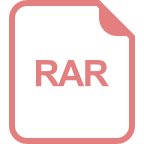
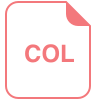
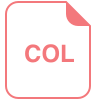
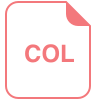
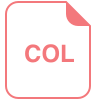
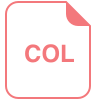









