Qt UDP接收端限制接收速度完整代码
时间: 2023-11-16 18:04:12 浏览: 124
以下是一个简单的 Qt UDP 接收端限制接收速度的完整代码示例:
```cpp
#include <QtWidgets>
#include <QUdpSocket>
class UdpReceiver : public QWidget
{
Q_OBJECT
public:
UdpReceiver(QWidget *parent = nullptr) : QWidget(parent)
{
setWindowTitle(tr("UDP Receiver"));
// 创建 UDP socket
udpSocket = new QUdpSocket(this);
// 绑定 UDP socket 到本地端口
udpSocket->bind(QHostAddress::Any, 1234);
// 设置接收缓冲区大小
udpSocket->setReadBufferSize(64 * 1024);
// 开始接收数据
connect(udpSocket, &QUdpSocket::readyRead, this, &UdpReceiver::processPendingDatagrams);
// 创建定时器,限制接收速度
receiveTimer = new QTimer(this);
receiveTimer->setInterval(1000); // 每秒接收一次数据
connect(receiveTimer, &QTimer::timeout, this, &UdpReceiver::startReceiving);
receiveTimer->start();
}
private slots:
void processPendingDatagrams()
{
while (udpSocket->hasPendingDatagrams())
{
QByteArray datagram;
datagram.resize(udpSocket->pendingDatagramSize());
udpSocket->readDatagram(datagram.data(), datagram.size());
// 处理接收到的数据
// ...
receivedBytes += datagram.size();
receivedDatagrams++;
}
}
void startReceiving()
{
// 停止接收数据
udpSocket->disconnectFromHost();
// 输出接收速率
qDebug() << "Received" << receivedBytes << "bytes in" << receivedDatagrams << "datagrams ("
<< receivedBytes / receiveTimer->interval() << " B/s)";
// 重置接收计数器
receivedBytes = 0;
receivedDatagrams = 0;
// 开始接收数据
udpSocket->connectToHost(QHostAddress::Any, 1234);
}
private:
QUdpSocket *udpSocket;
QTimer *receiveTimer;
qint64 receivedBytes = 0;
qint64 receivedDatagrams = 0;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
UdpReceiver receiver;
receiver.show();
return app.exec();
}
#include "main.moc"
```
此代码创建了一个 `QUdpSocket` 对象,并将其绑定到本地端口 `1234` 上。使用定时器限制接收速度,每秒最多接收一次数据。当接收到数据时,会输出接收速率,并重置接收计数器,以便下一个时间段内重新计算速率。
请注意,此示例中限制接收速度的方法是简单地断开并重新连接到 UDP 服务器,这可能会导致一些数据包丢失。更可靠的方法是在读取数据之前使用 `QUdpSocket::waitForReadyRead()` 等待足够的数据可用,以便在限制速率的同时保持接收所有数据。
阅读全文
相关推荐
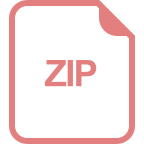
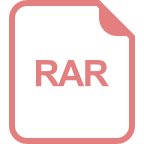
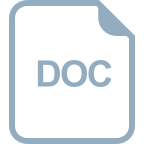


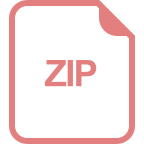
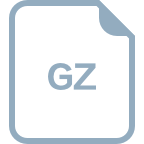
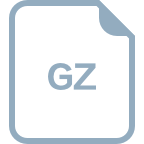
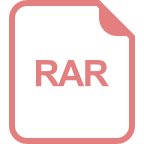
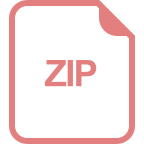
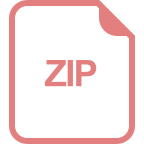
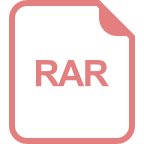
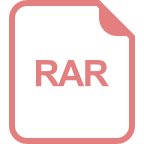
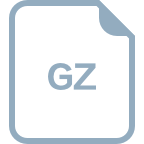



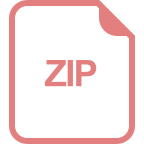