编写一个java设计一个栈类MyStack(元素为整数),包括pop、push、isEmpty、isFull、size等方法。构建栈对象时,如果不传递参数则栈的大小默认为10,否则按照参数的要求创建栈的大小。在测试类中构建不同大小的栈对象,并测试各成员函数的使用。
时间: 2024-10-23 17:16:27 浏览: 16
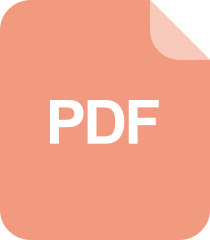
用C++实现一个链式栈的实例代码

在Java中,我们可以定义一个名为`MyStack`的自定义类,来模拟栈数据结构。以下是实现该类及其相关功能的方法:
```java
import java.util.EmptyStackException;
public class MyStack {
private int maxSize; // 栈的最大容量
private int top; // 栈顶指针
private int[] stackArray; // 存储栈元素
// 构造函数,如果传入了容量,则初始化大小;否则默认为10
public MyStack(int capacity) {
this.maxSize = capacity > 0 ? capacity : 10;
this.stackArray = new int[maxSize];
this.top = -1;
}
// push方法用于压栈
public void push(int value) throws StackOverflowError {
if (top == maxSize - 1) {
throw new StackOverflowError("Stack is full");
}
stackArray[++top] = value;
}
// pop方法用于出栈
public int pop() throws EmptyStackException {
if (isEmpty()) {
throw new EmptyStackException("Stack is empty");
}
return stackArray[top--];
}
// isEmpty检查栈是否为空
public boolean isEmpty() {
return top == -1;
}
// isFull检查栈是否已满
public boolean isFull() {
return top == maxSize - 1;
}
// size方法返回当前栈的元素数量
public int size() {
return top + 1;
}
}
// 测试类
public class MyStackTest {
public static void main(String[] args) {
// 创建一个默认大小为10的栈
MyStack smallStack = new MyStack();
System.out.println(smallStack.isEmpty()); // 输出true
smallStack.push(1);
smallStack.push(2);
System.out.println(smallStack.size()); // 输出2
System.out.println(smallStack.pop()); // 输出2
// 创建一个指定大小为5的栈
MyStack largeStack = new MyStack(5);
largeStack.push(3); // ... 推送更多元素并检查相应方法的行为
}
}
```
阅读全文
相关推荐
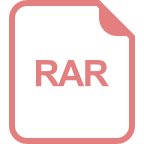
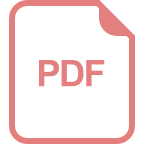






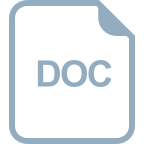







