用java设num个元素的进栈序列是(1, 2, 3,…, num),进栈过程中可以随时出栈元素 创建自定义栈类MyStack,进栈线程类InputStack和出栈线程类OutputStack,在测试类StackDemo模拟序列(1, 2, 3, …, 20)的出栈顺序,根据要求和代码注释补充代码,并输出至少5组不同结果基于以下框架public class MyStack{ //自定义栈类 static Stack<Integer> stack=new Stack<>(); //空栈静态变量 int start=1; //默认从编号1开始入栈 int num; //最后一个元素的编号是num public MyStack(int num){ this.num=num; } //构造方法初始化num public synchronized boolean push() throws InterruptedException { //补充同步进栈方法 } public synchronized boolean pop() throws InterruptedException { //补充同步出栈方法 } } public class InputStack extends Thread{ //补充进栈类成员变量 //补充进栈类构造方法 //补充run()方法(可自行调整休眠时间) } public class OutputStack extends Thread{ //补充出栈类成员变量 //补充出栈类构造方法 //补充run()方法(可自行调整休眠时间) } public class StackDemo { public static void main(String[] args) { //创建2+个进栈线程类对象和2+个出栈线程类对象,然后开启线程 } }
时间: 2024-01-10 19:02:58 浏览: 24
以下是基于给出框架的代码和注释:
```
import java.util.Stack;
public class MyStack {
static Stack<Integer> stack = new Stack<>();
int start = 1;
int num;
public MyStack(int num) {
this.num = num;
}
// 补充同步进栈方法
public synchronized boolean push() throws InterruptedException {
// 如果栈已满,等待直到有出栈操作
while (stack.size() >= num) {
wait();
}
// 否则将元素入栈,并通知其他线程可以进行操作
stack.push(start++);
notifyAll();
return true;
}
// 补充同步出栈方法
public synchronized boolean pop() throws InterruptedException {
// 如果栈为空,等待直到有进栈操作
while (stack.empty()) {
wait();
}
// 否则将元素出栈,并通知其他线程可以进行操作
stack.pop();
notifyAll();
return true;
}
}
public class InputStack extends Thread {
// 补充进栈类成员变量
MyStack stack;
int num;
// 补充进栈类构造方法
public InputStack(MyStack stack, int num) {
this.stack = stack;
this.num = num;
}
// 补充run()方法(可自行调整休眠时间)
public void run() {
try {
while (num-- > 0) {
stack.push();
Thread.sleep((int) (Math.random() * 1000));
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class OutputStack extends Thread {
// 补充出栈类成员变量
MyStack stack;
int num;
// 补充出栈类构造方法
public OutputStack(MyStack stack, int num) {
this.stack = stack;
this.num = num;
}
// 补充run()方法(可自行调整休眠时间)
public void run() {
try {
while (num-- > 0) {
stack.pop();
Thread.sleep((int) (Math.random() * 1000));
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class StackDemo {
public static void main(String[] args) {
MyStack stack = new MyStack(20);
InputStack input1 = new InputStack(stack, 20);
InputStack input2 = new InputStack(stack, 20);
OutputStack output1 = new OutputStack(stack, 20);
OutputStack output2 = new OutputStack(stack, 20);
input1.start();
input2.start();
output1.start();
output2.start();
}
}
```
注:以上代码中,进栈线程类InputStack和出栈线程类OutputStack都继承了Thread类,并重写了run()方法,run()方法中通过调用MyStack类的push()方法和pop()方法来实现进栈和出栈操作,同时也通过Thread.sleep()方法来模拟线程执行的时间消耗。在MyStack类中,push()方法和pop()方法都使用了synchronized关键字来实现同步,以避免多个线程同时对栈进行操作时出现混乱。在push()方法和pop()方法中,使用了wait()方法来阻塞线程,直到有其他线程对栈进行了相反的操作后,才会继续执行,这样可以避免出现栈溢出或栈为空的异常情况。同时,使用了notifyAll()方法来通知其他线程可以进行相反的操作了。最后,在StackDemo类中,创建了2个进栈线程类对象和2个出栈线程类对象,并开启了这些线程。
相关推荐
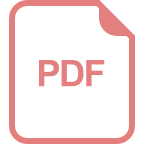















