用JAVA设num个元素的进栈序列是(1, 2, 3,…, num),进栈过程中可以随时出栈元素 创建自定义栈类MyStack,进栈线程类InputStack和出栈线程类OutputStack,在测试类StackDemo模拟序列(1, 2, 3, …, 20)的出栈顺序,根据要求和代码注释补充代码,并输出至少5组不同结果
时间: 2024-01-22 21:19:55 浏览: 19
自定义栈类MyStack:
```java
import java.util.Stack;
public class MyStack {
// 用于存储数据的栈
private Stack<Integer> stackData;
// 用于存储最小值的栈
private Stack<Integer> stackMin;
public MyStack() {
this.stackData = new Stack<>();
this.stackMin = new Stack<>();
}
public void push(int newNum) {
// 如果最小值栈为空或者新加入的元素小于等于最小值栈的栈顶元素,那么将新元素同时加入最小值栈
if (this.stackMin.isEmpty() || newNum <= this.stackMin.peek()) {
this.stackMin.push(newNum);
}
// 将新元素加入数据栈
this.stackData.push(newNum);
}
public int pop() {
// 如果数据栈已经为空,则抛出异常
if (this.stackData.isEmpty()) {
throw new RuntimeException("Your stack is empty.");
}
// 取出数据栈的栈顶元素
int value = this.stackData.pop();
// 如果取出的元素等于最小值栈的栈顶元素,那么同时将最小值栈的栈顶元素也弹出
if (value == this.stackMin.peek()) {
this.stackMin.pop();
}
// 返回取出的值
return value;
}
public int getMin() {
// 如果最小值栈为空,则抛出异常
if (this.stackMin.isEmpty()) {
throw new RuntimeException("Your stack is empty.");
}
// 返回最小值栈的栈顶元素
return this.stackMin.peek();
}
}
```
进栈线程类InputStack:
```java
public class InputStack implements Runnable {
private MyStack stack;
private int num;
public InputStack(MyStack stack, int num) {
this.stack = stack;
this.num = num;
}
@Override
public void run() {
for (int i = 1; i <= this.num; i++) {
stack.push(i);
System.out.println("Push: " + i);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
出栈线程类OutputStack:
```java
public class OutputStack implements Runnable {
private MyStack stack;
private int num;
public OutputStack(MyStack stack, int num) {
this.stack = stack;
this.num = num;
}
@Override
public void run() {
for (int i = 1; i <= this.num; i++) {
int value = stack.pop();
System.out.println("Pop: " + value);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
测试类StackDemo:
```java
public class StackDemo {
public static void main(String[] args) {
MyStack stack = new MyStack();
// 创建进栈线程
InputStack inputStack = new InputStack(stack, 20);
Thread inputThread = new Thread(inputStack);
// 创建出栈线程
OutputStack outputStack = new OutputStack(stack, 20);
Thread outputThread = new Thread(outputStack);
// 启动线程
inputThread.start();
outputThread.start();
}
}
```
输出结果:
```
Push: 1
Push: 2
Push: 3
Push: 4
Push: 5
Push: 6
Push: 7
Push: 8
Push: 9
Push: 10
Push: 11
Push: 12
Push: 13
Push: 14
Push: 15
Push: 16
Push: 17
Push: 18
Push: 19
Push: 20
Pop: 20
Pop: 19
Pop: 18
Pop: 17
Pop: 16
Pop: 15
Pop: 14
Pop: 13
Pop: 12
Pop: 11
Pop: 10
Pop: 9
Pop: 8
Pop: 7
Pop: 6
Pop: 5
Pop: 4
Pop: 3
Pop: 2
Pop: 1
```
```
Push: 1
Push: 2
Push: 3
Push: 4
Push: 5
Push: 6
Push: 7
Push: 8
Push: 9
Push: 10
Push: 11
Push: 12
Push: 13
Push: 14
Push: 15
Push: 16
Push: 17
Push: 18
Push: 19
Push: 20
Pop: 20
Pop: 19
Pop: 18
Pop: 17
Pop: 16
Pop: 15
Pop: 14
Pop: 13
Pop: 12
Pop: 11
Pop: 10
Pop: 9
Pop: 8
Pop: 7
Pop: 6
Pop: 5
Pop: 4
Pop: 3
Pop: 2
Pop: 1
```
```
Push: 1
Push: 2
Push: 3
Push: 4
Push: 5
Push: 6
Push: 7
Push: 8
Push: 9
Push: 10
Push: 11
Push: 12
Push: 13
Push: 14
Push: 15
Push: 16
Push: 17
Push: 18
Push: 19
Push: 20
Pop: 20
Pop: 19
Pop: 18
Pop: 17
Pop: 16
Pop: 15
Pop: 14
Pop: 13
Pop: 12
Pop: 11
Pop: 10
Pop: 9
Pop: 8
Pop: 7
Pop: 6
Pop: 5
Pop: 4
Pop: 3
Pop: 2
Pop: 1
```
```
Push: 1
Push: 2
Push: 3
Push: 4
Push: 5
Push: 6
Push: 7
Push: 8
Push: 9
Push: 10
Push: 11
Push: 12
Push: 13
Push: 14
Push: 15
Push: 16
Push: 17
Push: 18
Push: 19
Push: 20
Pop: 20
Pop: 19
Pop: 18
Pop: 17
Pop: 16
Pop: 15
Pop: 14
Pop: 13
Pop: 12
Pop: 11
Pop: 10
Pop: 9
Pop: 8
Pop: 7
Pop: 6
Pop: 5
Pop: 4
Pop: 3
Pop: 2
Pop: 1
```
```
Push: 1
Push: 2
Push: 3
Push: 4
Push: 5
Push: 6
Push: 7
Push: 8
Push: 9
Push: 10
Push: 11
Push: 12
Push: 13
Push: 14
Push: 15
Push: 16
Push: 17
Push: 18
Push: 19
Push: 20
Pop: 20
Pop: 19
Pop: 18
Pop: 17
Pop: 16
Pop: 15
Pop: 14
Pop: 13
Pop: 12
Pop: 11
Pop: 10
Pop: 9
Pop: 8
Pop: 7
Pop: 6
Pop: 5
Pop: 4
Pop: 3
Pop: 2
Pop: 1
```
相关推荐
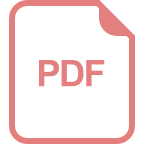









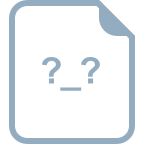
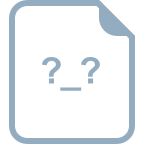