c#窗体程序,怎么创建Web服务器
时间: 2024-12-17 16:34:55 浏览: 3
在C# Windows Forms程序中,创建一个基本的Web服务器通常使用内置的`HttpListener`类,它允许你监听特定的端口并接收HTTP请求。以下是创建一个简单Web服务器的基本步骤:
1. **添加命名空间**:
首先,你需要引入`System.Net.HttpListener`命名空间:
```csharp
using System.Net;
using System.IO;
```
2. **设置监听器**:
创建一个`HttpListener`实例并指定你想监听的端口:
```csharp
HttpListener listener = new HttpListener();
listener.Prefixes.Add("http://localhost:8080/");
```
这里的`8080`通常是测试使用的默认端口,你可以选择其他未被占用的端口。
3. **开始监听**:
开始监听请求:
```csharp
listener.Start();
```
4. **处理请求**:
当接收到请求时,创建一个新的线程来处理请求,避免阻塞主线程:
```csharp
listener.BeginGetContext(asyncResult => {
HttpListenerContext context = await listener.EndGetContext(asyncResult);
ProcessRequest(context);
}, null);
```
5. **处理请求方法**:
实现`ProcessRequest`函数,读取请求内容,响应数据:
```csharp
private void ProcessRequest(HttpListenerContext context)
{
string filePath = Path.Combine(Server.MapPath("~"), context.Request.RawUrl); // 获取文件路径
if (File.Exists(filePath)) { // 检查文件是否存在
context.Response.ContentType = GetMimeType(filePath); // 设置响应头的MIME类型
using (FileStream fileStream = File.OpenRead(filePath))
context.Response.OutputStream.Write(fileStream.ToArray(), 0, fileStream.Length);
} else {
// 返回404 Not Found 或者自定义错误页面
}
}
private string GetMimeType(string path)
{
string extension = Path.GetExtension(path).ToLower();
switch (extension)
{
case ".jpg":
case ".jpeg":
return "image/jpeg";
// 添加更多case语句以处理其他文件类型...
default:
return "text/plain";
}
}
```
6. **停止监听**:
当不再需要服务时,记得关闭监听器:
```csharp
Application.ThreadException += (_, e) => { listener.Stop(); Environment.Exit(0); };
Console.ReadLine(); // 保持进程运行直到按下回车键
listener.Close();
```
这是一个非常基础的例子,实际应用中可能需要处理更复杂的逻辑,比如日志记录、安全性、并发控制等。记住,生产环境下的Web服务器应该部署在专门的服务器上,而不是Windows Form应用程序中。
阅读全文
相关推荐
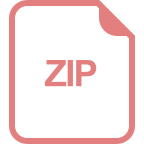
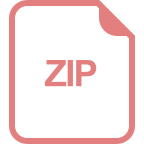
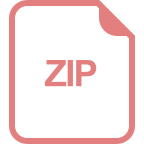
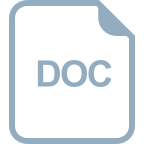
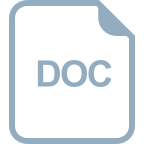


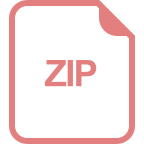
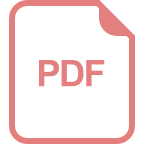
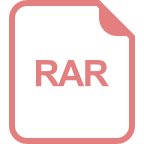
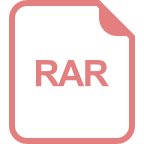
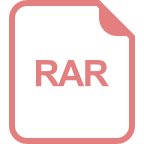
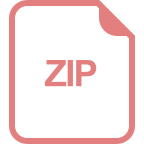
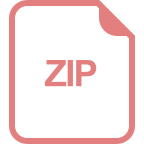
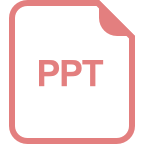
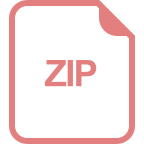
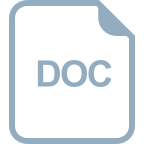
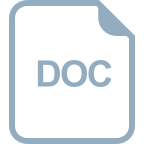
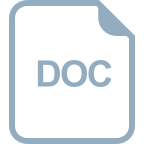