javacv实现rtsp转HTTP-FLV
时间: 2023-12-24 20:05:35 浏览: 69
使用 JavaCV 实现 RTSP 转 HTTP-FLV 需要用到 FFmpeg 和 Netty 的相关功能。以下是实现步骤:
1. 引入 JavaCV、FFmpeg 和 Netty 相关依赖:
```xml
<dependency>
<groupId>org.bytedeco</groupId>
<artifactId>javacv-platform</artifactId>
<version>1.5.6</version>
</dependency>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.63.Final</version>
</dependency>
<dependency>
<groupId>com.github.mhrimaz</groupId>
<artifactId>netty-http-flv</artifactId>
<version>1.0.1</version>
</dependency>
```
2. 创建 FFmpegFrameGrabber 和 FFmpegFrameRecorder,并设置相关参数:
```java
FFmpegFrameGrabber grabber = new FFmpegFrameGrabber("rtsp://example.com/stream");
grabber.setOption("rtsp_transport", "tcp"); // 使用 TCP 协议传输数据
grabber.start();
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder("http://localhost:8080/stream.flv", grabber.getImageWidth(), grabber.getImageHeight(), grabber.getAudioChannels());
recorder.setVideoCodec(avcodec.AV_CODEC_ID_H264); // 设置视频编码器为 H.264
recorder.setFormat("flv"); // 设置输出格式为 FLV
recorder.start();
```
3. 创建 Netty 的 HTTP-FLV 服务器,并将 FFmpegFrameRecorder 中的数据转发给客户端:
```java
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new HttpContentCompressor());
pipeline.addLast(new HttpFLVServerHandler(recorder));
}
});
ChannelFuture future = bootstrap.bind(8080).sync();
```
4. 循环读取 RTSP 数据,将数据转换为 FLV 格式并写入 FFmpegFrameRecorder 中:
```java
Frame frame = null;
while ((frame = grabber.grab()) != null) {
recorder.record(frame);
}
```
5. 释放资源:
```java
grabber.stop();
grabber.release();
recorder.stop();
recorder.release();
future.channel().closeFuture().sync();
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
```
以上是使用 JavaCV 实现 RTSP 转 HTTP-FLV 的基本步骤。需要注意的是,该方法需要使用 Netty 的 HTTP-FLV 服务器将数据转发给客户端,因此需要对 HTTP-FLV 相关技术有一定的了解。
相关推荐
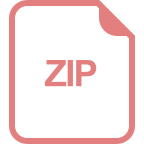














