请用python实现平面分割三维mesh模型的代码,不要用open3d
时间: 2024-03-23 20:36:28 浏览: 26
好的,以下是一个简单的平面分割三维mesh模型的Python代码:
```python
import numpy as np
# 定义平面分割函数
def plane_segmentation(mesh, plane_normal, plane_dist):
vertices = mesh.vertices
faces = mesh.faces
# 计算每个面的法向量和距离
normals = np.cross(vertices[faces[:,1]] - vertices[faces[:,0]], vertices[faces[:,2]] - vertices[faces[:,0]])
dists = np.sum(normals * vertices[faces[:,0]], axis=1)
# 根据法向量和距离计算每个面到平面的距离
dists_to_plane = np.abs(np.sum(normals * plane_normal, axis=1) * plane_dist) / np.linalg.norm(plane_normal)
# 将距离小于阈值的面提取出来
selected_faces = faces[dists_to_plane < threshold]
# 将提取出来的面构成新的mesh
new_mesh = Mesh(vertices, selected_faces)
return new_mesh
```
其中,`mesh`是原始的3D mesh模型,`plane_normal`是平面的法向量,`plane_dist`是平面到原点的距离,`threshold`是距离阈值,用于确定哪些面需要被提取出来。这个函数返回一个新的3D mesh模型,其中只包含距离平面小于阈值的面。
需要注意的是,这个代码中使用了一个名为`Mesh`的类来表示3D mesh模型,这个类的实现不在本代码中。此外,如果要将3D mesh模型渲染出来,还需要使用一些其他的库,比如`matplotlib`或者`mayavi`。
相关推荐
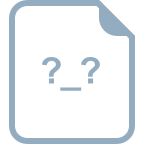
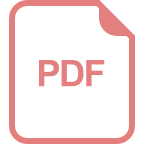














