用python代码实现用pyqt6将文件里面的图片通过图形界面一张一张显示出来,没过三秒每次只显示一张
时间: 2023-06-14 16:07:12 浏览: 193
可以使用PyQt6中的QTimer定时器和QLabel标签来实现这个功能。以下是一个简单的示例代码:
```python
import os
from PyQt6.QtCore import QTimer, Qt
from PyQt6.QtGui import QPixmap
from PyQt6.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout
class ImageViewer(QWidget):
def __init__(self):
super().__init__()
# 获取图片文件列表
self.image_files = []
for file in os.listdir('images'):
if file.endswith('.jpg') or file.endswith('.png'):
self.image_files.append(os.path.join('images', file))
# 设置标签
self.image_label = QLabel()
self.image_label.setAlignment(Qt.AlignmentFlag.AlignCenter)
# 设置布局
layout = QVBoxLayout()
layout.addWidget(self.image_label)
self.setLayout(layout)
# 设置定时器
self.timer = QTimer()
self.timer.timeout.connect(self.show_next_image)
self.timer.start(3000) # 每3秒切换一张图片
def show_next_image(self):
# 读取下一张图片
if len(self.image_files) > 0:
file = self.image_files.pop(0)
pixmap = QPixmap(file)
self.image_label.setPixmap(pixmap)
else:
self.timer.stop()
if __name__ == '__main__':
app = QApplication([])
viewer = ImageViewer()
viewer.show()
app.exec()
```
在这个例子中,我们首先获取指定文件夹中的所有图片文件,并将它们存储在self.image_files列表中。然后,我们创建一个QLabel标签,并将其添加到垂直布局中。接下来,我们设置一个QTimer定时器,并将其连接到show_next_image方法上。每次定时器超时时,该方法将从self.image_files列表中获取下一张图片并将其显示在QLabel中。如果self.image_files列表为空,则停止定时器。最后,我们创建一个QApplication实例和一个ImageViewer实例,并显示它们。
需要注意的是,这个例子假设所有的图片都可以用QPixmap类来加载。如果你需要加载其他类型的图片文件,请使用适当的Qt图像类来代替QPixmap。
阅读全文
相关推荐
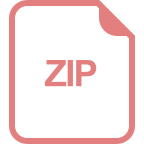
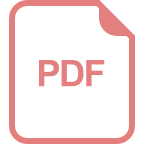


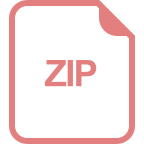
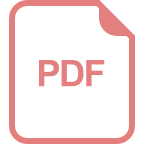
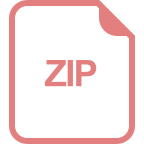
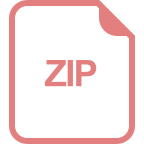
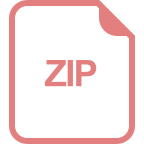
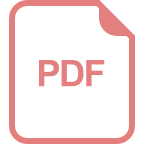
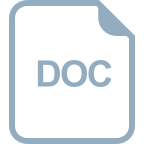
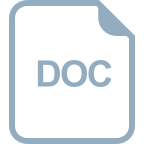
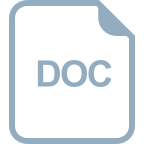
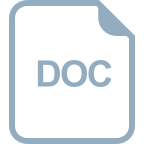
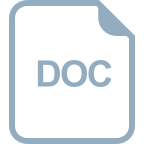

