用python代码实现用pyqt6不用qtgui将文件里面的图片通过图形界面一张一张显示出来,没过三秒每次只显示一张
时间: 2023-06-14 11:07:11 浏览: 83
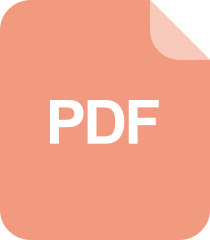
python如何实现读取并显示图片(不需要图形界面)
可以使用QTimer来实现定时器,每隔三秒钟更新一张图片。
以下是实现代码:
```python
import sys
from PyQt6.QtWidgets import QApplication, QMainWindow, QLabel
from PyQt6.QtGui import QPixmap
from PyQt6.QtCore import QTimer
class ImageDisplay(QMainWindow):
def __init__(self, file_list):
super().__init__()
self.file_list = file_list
self.current_index = 0
self.label = QLabel(self)
self.label.setGeometry(50, 50, 400, 400)
self.timer = QTimer(self)
self.timer.timeout.connect(self.show_image)
self.timer.start(3000)
def show_image(self):
if self.current_index >= len(self.file_list):
self.timer.stop()
return
pixmap = QPixmap(self.file_list[self.current_index])
self.label.setPixmap(pixmap.scaled(self.label.width(), self.label.height()))
self.current_index += 1
if __name__ == '__main__':
app = QApplication(sys.argv)
file_list = ["image1.jpg", "image2.jpg", "image3.jpg"]
window = ImageDisplay(file_list)
window.show()
sys.exit(app.exec())
```
其中,`file_list`是图片文件名列表,`current_index`表示当前显示的图片索引,`label`是用来显示图片的QLabel控件。
在`__init__`函数中,首先初始化QLabel控件和QTimer对象,然后通过`timer.timeout.connect`将定时器的timeout信号连接到`show_image`函数。最后,启动定时器。
在`show_image`函数中,如果当前索引超出了图片文件名列表的长度,则停止定时器。否则,读取当前索引对应的图片文件,将其转换为QPixmap对象,并将其显示在QLabel控件上。最后,将当前索引加1。
运行程序后,每隔三秒钟就会显示一张图片,直到所有图片都被显示完。
阅读全文
相关推荐

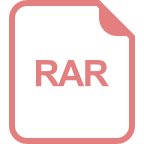
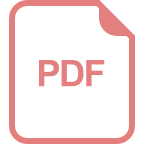
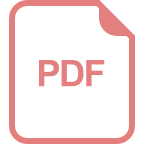
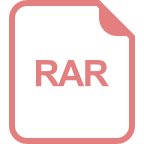
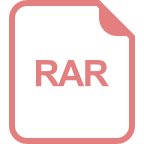
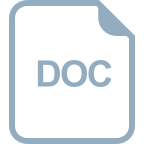
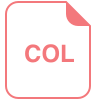




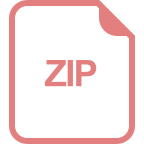
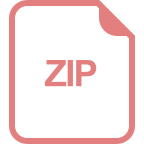
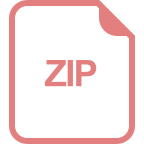