判断密码强度,数字、小写字母、大写字母和指定的标点符号,分别对应 weak、 below middle、above middle、strong。python
时间: 2024-01-26 07:02:53 浏览: 31
可以使用正则表达式来实现密码强度的判断,以下是示例代码:
```python
import re
def check_password_strength(password):
# 匹配数字、小写字母、大写字母和指定的标点符号
digit_pattern = re.compile(r'\d')
lowercase_pattern = re.compile(r'[a-z]')
uppercase_pattern = re.compile(r'[A-Z]')
symbol_pattern = re.compile(r'[\W_]')
# 计算密码中包含的不同字符类型的数量
digit_count = len(digit_pattern.findall(password))
lowercase_count = len(lowercase_pattern.findall(password))
uppercase_count = len(uppercase_pattern.findall(password))
symbol_count = len(symbol_pattern.findall(password))
# 根据不同字符类型数量的多少判断密码强度等级
if len(password) < 8 or (digit_count == 0 and lowercase_count == 0 and uppercase_count == 0 and symbol_count == 0):
return 'weak'
elif (digit_count > 0 and lowercase_count > 0 and uppercase_count > 0 and symbol_count > 0) or len(password) >= 12:
return 'strong'
else:
return 'above middle' if len(password) >= 10 else 'below middle'
```
可以通过调用 `check_password_strength` 函数并传入密码来获取密码的强度等级。例如:
```python
password = '123456'
strength = check_password_strength(password)
print(strength) # 输出 weak
```
相关推荐
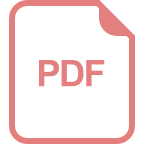
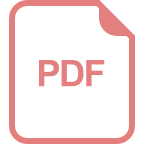
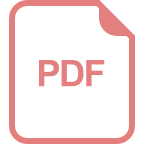














