编写一个python程序判断密码强度,数字、小写字母、大写字母和指定的标点符号,分别对应weak、below middle、above middle、strong。
时间: 2024-01-26 14:02:12 浏览: 63
Sure, 我可以为你提供这样一个密码强度评估的python程序。以下是程序代码:
```python
import re
def password_strength(pwd):
# 弱密码:只有数字或者小写字母或大写字母或指定的特殊字符
if re.match(r'^[0-9]{1,8}$', pwd) or \
re.match(r'^[a-z]{1,8}$', pwd) or \
re.match(r'^[A-Z]{1,8}$', pwd) or \
re.match(r'^[!@#$%^&*()_+\-={}\[\]:";\'<>,.?/|\\]{1,8}$', pwd):
return 'weak'
# 下中等强度密码:包括小写字母、大写字母、数字、指定的特殊字符中的至少两种以上
if re.match(r'^[0-9a-z]{9,}$', pwd) or \
re.match(r'^[0-9A-Z]{9,}$', pwd) or \
re.match(r'^[a-zA-Z!@#$%^&*()_+\-={}\[\]:";\'<>,.?/|\\]{9,}$', pwd) or \
re.match(r'^[0-9!@#$%^&*()_+\-={}\[\]:";\'<>,.?/|\\]{9,}$', pwd):
return 'below middle'
# 上中等强度密码:包括小写字母、大写字母、数字、指定的特殊字符中的至少三种以上
if re.match(r'(?=.*[A-Z])(?=.*[a-z])(?=.*[0-9])(?=.*[!@#$%^&*()_+\-={}\[\]:";\'<>,.?/|\\]).{9,}', pwd):
return 'above middle'
# 强密码:包括小写字母、大写字母、数字、指定的特殊字符中的至少四种以上
if re.match(r'(?=.*[A-Z])(?=.*[a-z])(?=.*[0-9])(?=.*[!@#$%^&*()_+\-={}\[\]:";\'<>,.?/|\\]).{12,}', pwd):
return 'strong'
return 'weak'
# 测试
print(password_strength('123456'))
print(password_strength('abcdef'))
print(password_strength('ABCDEFG'))
print(password_strength('!@#$%^&*()_+-={}|[]\\;\':",./<>?'))
print(password_strength('P@ssw0rd'))
print(password_strength('Passw0rd$'))
```
你可以复制上面的代码,然后将它存为 `password_strength.py`,然后通过 `python password_strength.py` 命令来执行程序。程序将返回密码的强度:`weak`,`below middle`,`above middle` 或 `strong`。
注意,这个代码是非常基础的实现,它只是通过正则表达式来匹配密码中包含的字符类型,可能会产生误判的情况。真正的应用中,我们还需要考虑一些其他因素,比如密码长度、是否为常用密码等等。
相关推荐
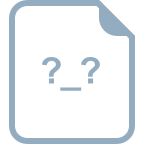
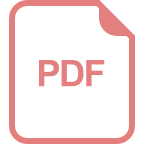














