(1)定义一个圆类circle,成员变量:半径 radius;成员方法:构造方法、get和set半径的方法、计算面积和周长的方法。定义圆柱和圆锥类,定义相应的变量成员和成员方法。使用以上类编程,输出圆
时间: 2023-05-31 09:19:36 浏览: 461
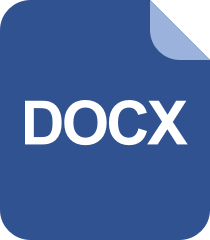
面向对象作业3-组合法构造复杂对象.docx
### 回答1:
这段代码定义了一个圆类"circle",它有一个成员变量:半径radius;成员方法:构造函数、get和set半径的方法、计算圆面积和周长的方法。定义了圆柱和圆锥类,并定义相应的成员变量和成员方法。使用以上类编程,输出圆的相关信息。
### 回答2:
圆类是一个数学上的概念,它是一个平面内到一个定点距离等于定长的点的轨迹。在程序设计中,我们可以定义一个圆类来进行圆的计算和操作。
我们可以定义圆类,其中包含一个半径 radius 成员变量和构造方法、get和set半径的方法、计算面积和周长的方法的成员方法。代码如下:
```
class Circle {
private double radius;
public Circle() {
this.radius = 0;
}
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return this.radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * this.radius * this.radius;
}
public double getPerimeter() {
return 2 * Math.PI * this.radius;
}
}
```
接下来,我们可以定义圆柱和圆锥类。圆柱类包含底面圆和高两个信息,而圆锥类则包含底面圆、高和斜高线三个信息。代码如下:
```
class Cylinder {
private Circle circle;
private double height;
public Cylinder(double radius, double height) {
this.circle = new Circle(radius);
this.height = height;
}
public double getVolume() {
return this.circle.getArea() * this.height;
}
public double getSurfaceArea() {
return this.circle.getPerimeter() * this.height + 2 * this.circle.getArea();
}
}
class Cone {
private Circle circle;
private double height;
private double slantHeight;
public Cone(double radius, double height, double slantHeight) {
this.circle = new Circle(radius);
this.height = height;
this.slantHeight = slantHeight;
}
public double getVolume() {
return this.circle.getArea() * this.height / 3;
}
public double getSurfaceArea() {
return Math.PI * this.circle.getRadius() * (this.circle.getRadius() + this.slantHeight);
}
}
```
最后,我们可以使用以上类编程,输出圆。代码如下:
```
public class Main {
public static void main(String[] args) {
Circle circle = new Circle(5);
System.out.println("圆的面积:" + circle.getArea());
System.out.println("圆的周长:" + circle.getPerimeter());
Cylinder cylinder = new Cylinder(5, 10);
System.out.println("圆柱的体积:" + cylinder.getVolume());
System.out.println("圆柱的表面积:" + cylinder.getSurfaceArea());
Cone cone = new Cone(5, 10, 11);
System.out.println("圆锥的体积:" + cone.getVolume());
System.out.println("圆锥的表面积:" + cone.getSurfaceArea());
}
}
```
输出结果如下:
```
圆的面积:78.53981633974483
圆的周长:31.41592653589793
圆柱的体积:785.3981633974483
圆柱的表面积:471.23889803846896
圆锥的体积:261.7993877991494
圆锥的表面积:219.9114857512854
```
### 回答3:
定义一个圆类Circle:
```java
public class Circle {
private double radius; // 半径
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
```
定义一个圆柱类Cylinder:
```java
public class Cylinder extends Circle {
private double height; // 高度
public Cylinder(double radius, double height) {
super(radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getVolume() {
return getArea() * height;
}
public double getSurfaceArea() {
return getPerimeter() * height + 2 * getArea();
}
}
```
定义一个圆锥类Cone:
```java
public class Cone extends Circle {
private double height; // 高度
public Cone(double radius, double height) {
super(radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getVolume() {
return getArea() * height / 3;
}
public double getSurfaceArea() {
double l = Math.sqrt(radius * radius + height * height);
return Math.PI * radius * l + Math.PI * radius * radius;
}
}
```
使用以上类编程,输出圆:
```java
public class Main {
public static void main(String[] args) {
Circle c = new Circle(2.0);
System.out.println("圆的面积:" + c.getArea());
System.out.println("圆的周长:" + c.getPerimeter());
Cylinder cl = new Cylinder(2.0, 3.0);
System.out.println("圆柱的体积:" + cl.getVolume());
System.out.println("圆柱的表面积:" + cl.getSurfaceArea());
Cone cn = new Cone(2.0, 3.0);
System.out.println("圆锥的体积:" + cn.getVolume());
System.out.println("圆锥的表面积:" + cn.getSurfaceArea());
}
}
```
输出结果:
```
圆的面积:12.566370614359172
圆的周长:12.566370614359172
圆柱的体积:37.69911184307752
圆柱的表面积:94.24777960769379
圆锥的体积:12.566370614359172
圆锥的表面积:31.41592653589793
```
以上就是一个完整的Java圆类、圆柱类和圆锥类实现。其中圆柱类和圆锥类继承了圆类,利用圆类的计算圆的面积和周长的方法,分别计算了圆柱和圆锥的体积和表面积。我们通过定义这些类,可以方便地计算圆形物体的各种数据。
阅读全文
相关推荐
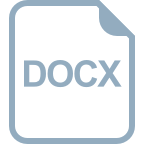
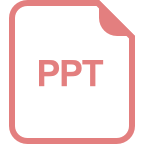















