python模拟学生选课
时间: 2023-10-22 11:16:42 浏览: 143
好的,让我们来模拟一个学生选课的程序吧!
首先,我们需要定义一些课程和学生对象。我们可以用类来表示它们。每个课程有一个名称和一个容量,每个学生有一个姓名和一个已选课程的列表。
```python
class Course:
def __init__(self, name, capacity):
self.name = name
self.capacity = capacity
self.students = []
def add_student(self, student):
if len(self.students) < self.capacity:
self.students.append(student)
return True
else:
return False
class Student:
def __init__(self, name):
self.name = name
self.courses = []
def add_course(self, course):
self.courses.append(course)
```
接下来,我们可以定义一些课程和学生。
```python
math = Course("Math", 2)
science = Course("Science", 1)
history = Course("History", 3)
john = Student("John")
mary = Student("Mary")
```
现在我们有了一些课程和学生,我们需要实现一个选课函数,使学生可以选择他们想要的课程。
```python
def enroll(student, course):
if course.add_student(student):
student.add_course(course)
print(f"{student.name} has successfully enrolled in {course.name}.")
else:
print(f"Sorry, {course.name} is full. {student.name} cannot enroll in this course.")
```
最后,我们可以让学生选择他们想要的课程。
```python
enroll(john, math) # John has successfully enrolled in Math.
enroll(mary, science) # Mary has successfully enrolled in Science.
enroll(john, science) # Sorry, Science is full. John cannot enroll in this course.
enroll(mary, math) # Sorry, Math is full. Mary cannot enroll in this course.
enroll(john, history) # John has successfully enrolled in History.
enroll(mary, history) # Mary has successfully enrolled in History.
```
现在,我们的程序就可以模拟学生选课了!
阅读全文
相关推荐
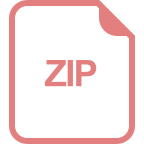




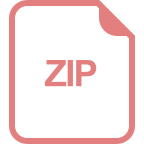
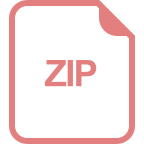
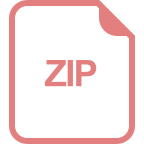
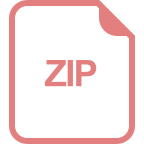
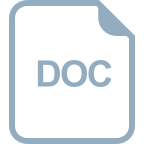
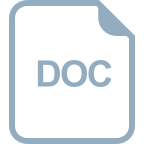
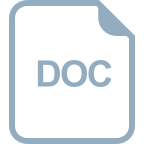



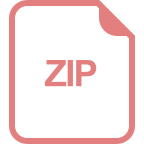