模拟学生选课,每选一门课程,更新科目和学分,将课程信息加入到学生所选的课程信息中,同时将学分累加到总学分中
时间: 2024-05-03 12:17:31 浏览: 10
好的,这是一个简单的模拟学生选课的程序:
```python
class Course:
def __init__(self, name, credit):
self.name = name
self.credit = credit
class Student:
def __init__(self, name):
self.name = name
self.courses = []
self.total_credit = 0
def select_course(self, course):
self.courses.append(course)
self.total_credit += course.credit
print(f"{self.name} has selected course {course.name} ({course.credit} credits).")
print(f"Total credits: {self.total_credit}")
def drop_course(self, course):
if course in self.courses:
self.courses.remove(course)
self.total_credit -= course.credit
print(f"{self.name} has dropped course {course.name} ({course.credit} credits).")
print(f"Total credits: {self.total_credit}")
else:
print(f"{self.name} is not enrolled in course {course.name}.")
```
这里定义了两个类,`Course` 表示一门课程,包括名称和学分;`Student` 表示一个学生,包括姓名、所选课程列表、总学分。`select_course` 方法表示选课,接收一个 `Course` 对象作为参数,将该课程加入到学生所选的课程列表中,并累加学分。`drop_course` 方法表示退课,接收一个 `Course` 对象作为参数,将该课程从学生所选的课程列表中删除,并减去学分。
使用示例:
```python
# 创建一些课程和学生
math = Course("Math", 3)
english = Course("English", 2)
physics = Course("Physics", 4)
alice = Student("Alice")
bob = Student("Bob")
# Alice 选课
alice.select_course(math)
alice.select_course(english)
alice.select_course(physics)
# Bob 选课
bob.select_course(math)
bob.select_course(physics)
# Alice 退课
alice.drop_course(english)
```
输出:
```
Alice has selected course Math (3 credits).
Total credits: 3
Alice has selected course English (2 credits).
Total credits: 5
Alice has selected course Physics (4 credits).
Total credits: 9
Bob has selected course Math (3 credits).
Total credits: 3
Bob has selected course Physics (4 credits).
Total credits: 7
Alice has dropped course English (2 credits).
Total credits: 7
```
相关推荐
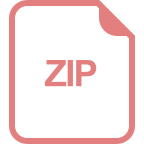
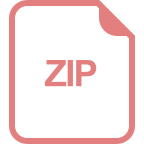
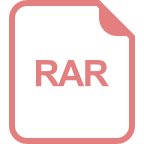














