C语言实现设计一款俄罗斯方块游戏
时间: 2023-12-11 18:04:33 浏览: 137
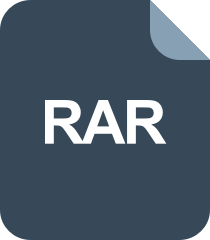
基于c语言的俄罗斯方块游戏设计与代码实现
好的,下面是一个简单的C语言实现俄罗斯方块游戏的代码。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#define MAX_X 10
#define MAX_Y 20
int board[MAX_X][MAX_Y] = {0};
int block[7][4][4] = {
// I型方块
{
{0, 0, 0, 0},
{1, 1, 1, 1},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// J型方块
{
{1, 0, 0, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// L型方块
{
{0, 0, 1, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// O型方块
{
{1, 1, 0, 0},
{1, 1, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// S型方块
{
{0, 1, 1, 0},
{1, 1, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// T型方块
{
{0, 1, 0, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// Z型方块
{
{1, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
}
};
int block_x = 0;
int block_y = 0;
int block_type = 0;
void init() {
srand((unsigned)time(NULL));
block_type = rand() % 7;
block_x = MAX_X / 2 - 2;
block_y = 0;
}
void show() {
system("cls");
for (int y = 0; y < MAX_Y; y++) {
for (int x = 0; x < MAX_X; x++) {
if (board[x][y] == 1) {
printf("*");
} else if (x >= block_x && x < block_x + 4 && y >= block_y && y < block_y + 4 && block[block_type][y - block_y][x - block_x] == 1) {
printf("*");
} else {
printf(" ");
}
}
printf("\n");
}
}
int check(int x, int y, int type) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[type][i][j] == 1) {
int nx = x + j;
int ny = y + i;
if (nx < 0 || nx >= MAX_X || ny >= MAX_Y) {
return 0;
}
if (ny >= 0 && board[nx][ny] == 1) {
return 0;
}
}
}
}
return 1;
}
void move(int dx, int dy) {
if (check(block_x + dx, block_y + dy, block_type)) {
block_x += dx;
block_y += dy;
}
}
void rotate() {
int next_type = (block_type + 1) % 7;
if (check(block_x, block_y, next_type)) {
block_type = next_type;
}
}
void update() {
if (check(block_x, block_y + 1, block_type)) {
block_y++;
} else {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[block_type][i][j] == 1) {
board[block_x + j][block_y + i] = 1;
}
}
}
init();
}
}
int main() {
init();
while (1) {
show();
char c = getch();
switch (c) {
case 'a':
move(-1, 0);
break;
case 'd':
move(1, 0);
break;
case 's':
update();
break;
case 'w':
rotate();
break;
case 'q':
exit(0);
break;
}
}
return 0;
}
```
这个代码使用了一个二维数组来表示游戏面板,另外还定义了七种不同类型的方块,以及当前正在掉落的方块的位置和类型。
在 `init` 函数中生成随机的方块类型,并将当前正在掉落的方块的初始位置设置为游戏面板的中心位置。
在 `show` 函数中,使用 `printf` 函数打印出游戏面板和方块。
在 `check` 函数中,检查方块是否会与已经落下的方块或者游戏面板的边界碰撞。
在 `move` 函数中,如果方块可以向左或者向右移动,则更新方块的位置。
在 `rotate` 函数中,如果方块可以旋转,则更新方块的类型。
在 `update` 函数中,如果方块已经到达底部,则将方块加入到已经落下的方块中,并生成新的方块。
在 `main` 函数中,使用 `getch` 函数获取用户输入的指令,并根据指令更新方块的位置和类型,最终实现俄罗斯方块游戏的功能。
阅读全文
相关推荐
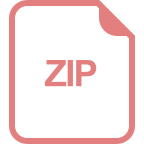
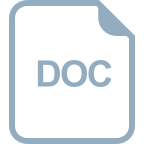
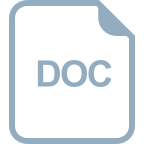
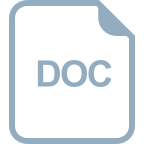
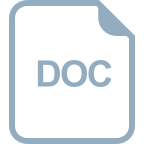
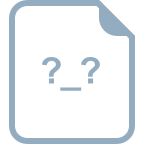
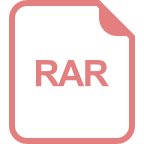
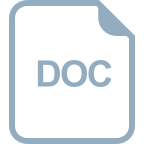
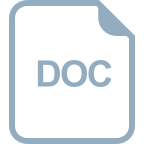
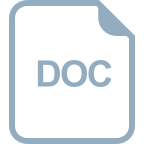
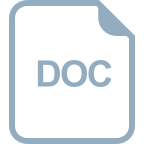
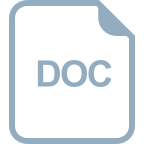
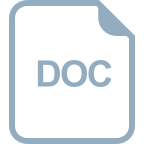
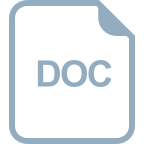
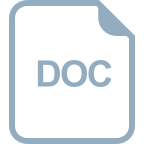
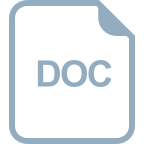
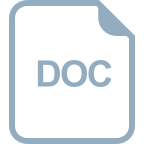