要求: 基于JSP+JavaBean的设计模式,设计任意两个复数实现4则运算(复数加法、减法、乘法、除法)的Web程序
时间: 2023-12-17 10:03:53 浏览: 171
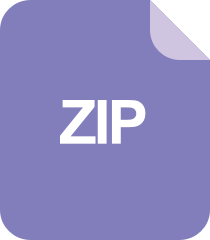
基于jsp+Javabean+servlet实现的创新驿站系统.zip
好的,这里提供一种基于JSP+JavaBean的设计模式,实现复数四则运算的Web程序的设计思路。
1. 设计复数类
首先,我们需要设计一个复数类,包含复数的实部和虚部两个属性,以及加法、减法、乘法、除法四个方法。代码如下:
```java
public class Complex {
private double real; // 实部
private double imag; // 虚部
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public double getReal() {
return real;
}
public double getImag() {
return imag;
}
public Complex add(Complex other) {
double real = this.real + other.real;
double imag = this.imag + other.imag;
return new Complex(real, imag);
}
public Complex subtract(Complex other) {
double real = this.real - other.real;
double imag = this.imag - other.imag;
return new Complex(real, imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
}
```
2. 设计JavaBean
接下来,我们需要设计一个JavaBean,用于实现Web页面与后台Java代码的交互。该JavaBean应该包含两个复数对象和一个运算符属性,以及相应的get和set方法。代码如下:
```java
public class CalculatorBean {
private Complex num1;
private Complex num2;
private String operator;
public Complex getNum1() {
return num1;
}
public void setNum1(Complex num1) {
this.num1 = num1;
}
public Complex getNum2() {
return num2;
}
public void setNum2(Complex num2) {
this.num2 = num2;
}
public String getOperator() {
return operator;
}
public void setOperator(String operator) {
this.operator = operator;
}
public Complex getResult() {
switch (operator) {
case "+":
return num1.add(num2);
case "-":
return num1.subtract(num2);
case "*":
return num1.multiply(num2);
case "/":
return num1.divide(num2);
default:
return null;
}
}
}
```
3. 设计JSP页面
最后,我们需要设计一个JSP页面,用于显示Web页面和用户输入,以及调用JavaBean中的方法进行计算。代码如下:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="com.example.calculator.Complex" %>
<%@ page import="com.example.calculator.CalculatorBean" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>复数计算器</title>
</head>
<body>
<h1>复数计算器</h1>
<form method="post" action="calculator.jsp">
<input type="text" name="num1.real"> + <input type="text" name="num1.imag">i
<select name="operator">
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select>
<input type="text" name="num2.real"> + <input type="text" name="num2.imag">i
<input type="submit" value="计算">
</form>
<%
CalculatorBean bean = new CalculatorBean();
bean.setNum1(new Complex(Double.parseDouble(request.getParameter("num1.real")),
Double.parseDouble(request.getParameter("num1.imag"))));
bean.setNum2(new Complex(Double.parseDouble(request.getParameter("num2.real")),
Double.parseDouble(request.getParameter("num2.imag"))));
bean.setOperator(request.getParameter("operator"));
Complex result = bean.getResult();
if (result != null) {
out.println(bean.getNum1().getReal() + "+" + bean.getNum1().getImag() + "i "
+ bean.getOperator() + " "
+ bean.getNum2().getReal() + "+" + bean.getNum2().getImag() + "i = "
+ result.getReal() + "+" + result.getImag() + "i");
}
%>
</body>
</html>
```
以上就是基于JSP+JavaBean的设计模式,实现复数四则运算的Web程序的设计思路。通过这种设计模式,我们可以实现页面与后台代码的解耦,方便维护和扩展。
阅读全文
相关推荐
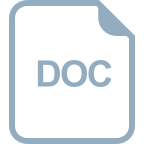
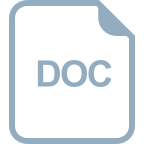
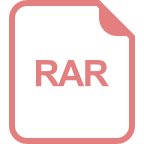
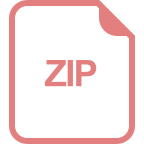
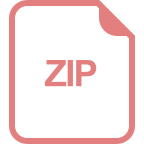
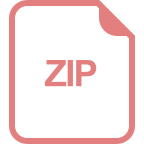
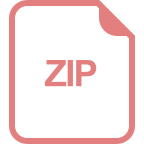
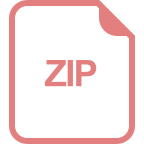
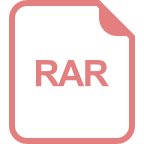
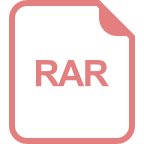
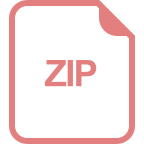
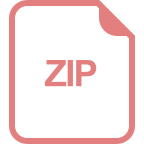
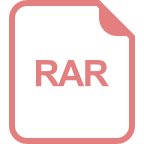
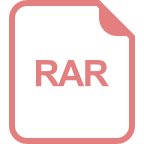
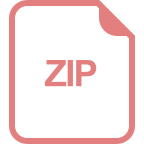
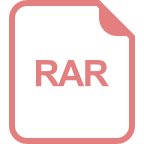
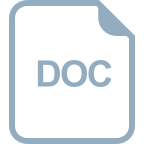