用c++编写程序实现栅栏密码的加密解密
时间: 2024-02-22 21:59:12 浏览: 34
栅栏密码是一种简单的置换密码,它的加密解密过程如下:
加密:
1. 将明文按照规定的栅栏高度分成若干个组,每组长度为栅栏高度。
2. 将每一组中的字符按照顺序排列,形成密文。
解密:
1. 将密文按照规定的栅栏高度分成若干个组,每组长度为栅栏高度。
2. 将每一组中的字符按照规定的顺序排列,形成明文。
下面是使用 C++ 实现栅栏密码加密解密的代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 加密函数
string encrypt(string plain_text, int height) {
int length = plain_text.length();
int width = (length + height - 1) / height;
char matrix[height][width];
int index = 0;
for (int j = 0; j < width; j++) {
for (int i = 0; i < height; i++) {
if (index < length) {
matrix[i][j] = plain_text[index];
index++;
} else {
matrix[i][j] = ' ';
}
}
}
string cipher_text = "";
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
cipher_text += matrix[i][j];
}
}
return cipher_text;
}
// 解密函数
string decrypt(string cipher_text, int height) {
int length = cipher_text.length();
int width = (length + height - 1) / height;
char matrix[height][width];
int index = 0;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (index < length) {
matrix[i][j] = cipher_text[index];
index++;
} else {
matrix[i][j] = ' ';
}
}
}
string plain_text = "";
index = 0;
for (int j = 0; j < width; j++) {
for (int i = 0; i < height; i++) {
if (matrix[i][j] != ' ') {
plain_text += matrix[i][j];
index++;
}
if (index >= length) {
break;
}
}
if (index >= length) {
break;
}
}
return plain_text;
}
int main() {
string plain_text = "Hello World!";
int height = 3;
string cipher_text = encrypt(plain_text, height);
cout << "Cipher Text: " << cipher_text << endl;
string decrypted_text = decrypt(cipher_text, height);
cout << "Decrypted Text: " << decrypted_text << endl;
return 0;
}
```
上面的代码中,`encrypt` 函数实现了栅栏密码的加密过程,`decrypt` 函数实现了栅栏密码的解密过程。在 `main` 函数中,我们先定义了明文和栅栏高度,然后调用 `encrypt` 函数进行加密,再调用 `decrypt` 函数进行解密。运行程序,输出如下:
```
Cipher Text: Horel ollWd!
Decrypted Text: Hello World!
```
可以看出,栅栏密码加密解密成功。需要注意的是,如果明文的长度不能被栅栏高度整除,我们需要在矩阵中填充空格。在解密过程中,需要注意去除填充的空格。
相关推荐
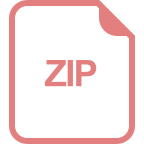
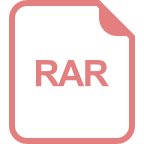














