栅栏密码C++字母明文密文详细代码
时间: 2024-10-15 21:08:54 浏览: 55
栅栏密码(Fence or Vigenère Cipher),也被称为维吉尼亚密码(Vigenère Cipher),是一种简单的替换式加密技术,通过将明文按照一定的规则分组,并用字母表中的字符进行位移来加密。在C++中实现一个简单的栅栏密码,我们可以创建一个函数接受明文、密钥和行数作为输入,然后生成密文。
下面是一个基础的栅栏密码加密和解密的C++代码示例:
```cpp
#include <iostream>
#include <string>
std::string encrypt(const std::string& plaintext, const std::string& key, int rows) {
std::string ciphertext = "";
for (int i = 0; i < plaintext.length(); ++i) {
if (rows > 0) {
int offset = key[i % key.length()] - 'A';
ciphertext += static_cast<char>((plaintext[i] - 'A' + offset) % 26 + 'A');
} else {
ciphertext += plaintext[i];
}
}
return ciphertext;
}
std::string decrypt(const std::string& ciphertext, const std::string& key, int rows) {
if (rows == 0) {
// 如果是解密,行数设为负数表示行数相反
rows = -rows;
}
return encrypt(ciphertext, key, rows);
}
int main() {
std::string plaintext = "Hello, World!";
std::string key = "KEY";
int rows = 3;
std::string encrypted_text = encrypt(plaintext, key, rows);
std::cout << "Encrypted Text: " << encrypted_text << std::endl;
std::string decrypted_text = decrypt(encrypted_text, key, rows);
std::cout << "Decrypted Text: " << decrypted_text << std::endl;
return 0;
}
```
在这个代码中,`encrypt`函数负责加密,`decrypt`函数负责解密。注意,这个版本只适用于小写字母的英文文本,如果需要处理其他字符集,你需要对算法稍作调整。
阅读全文
相关推荐
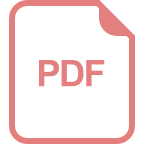
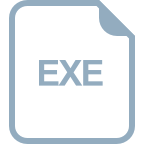
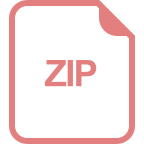
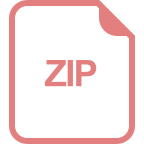
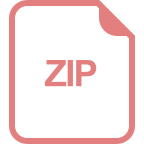
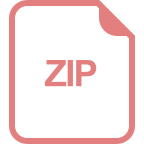
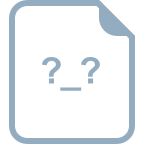
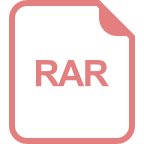
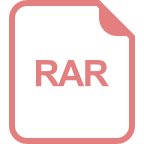
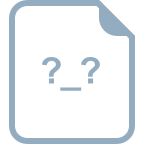
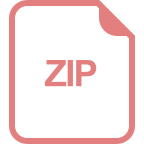
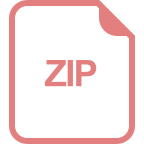
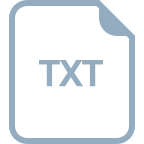
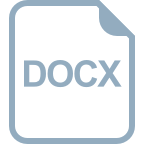
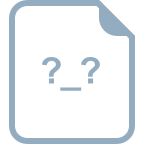
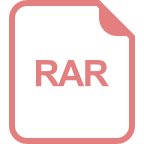