用c++语言写链栈实现数制的转换(十进制转八进制)的程序
时间: 2024-10-13 21:18:05 浏览: 70
在C++中,我们可以使用链表数据结构来实现一个简单的链栈,并利用它来辅助数制转换。这里是一个基本的示例,展示如何将十进制整数转换成八进制:
```cpp
#include <iostream>
#include <string>
// 定义节点结构体
struct Node {
int value;
Node* next; // 指向下一个节点的指针
};
// 链栈类,包含入栈、出栈操作
class Stack {
private:
Node* top;
public:
Stack() { top = nullptr; }
~Stack() { clear(); }
void push(int val) {
Node* newNode = new Node();
newNode->value = val;
newNode->next = top;
top = newNode;
}
int pop() {
if (top == nullptr) {
throw std::runtime_error("Stack is empty");
}
int ret = top->value;
Node* temp = top;
top = top->next;
delete temp;
return ret;
}
bool isEmpty() const { return top == nullptr; }
void clear() {
Node* current = top;
while (current != nullptr) {
Node* temp = current;
current = current->next;
delete temp;
}
top = nullptr;
}
};
// 十进制转八进制
std::string decimalToOctal(int decimal) {
Stack stack;
while (decimal > 0) {
stack.push(decimal % 8);
decimal /= 8;
}
std::string octal = "";
while (!stack.isEmpty()) {
octal += char('0' + stack.pop());
}
return octal;
}
int main() {
try {
int decimalNum = 12345;
std::string octalNum = decimalToOctal(decimalNum);
std::cout << "Decimal: " << decimalNum << "\n";
std::cout << "Octal: " << octalNum << "\n";
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
这个程序首先创建了一个链栈,然后不断地从十进制数值中取出余数并压入栈,直到十进制数变为0。最后,栈顶的元素就是对应的八进制位,依次读取并拼接成字符串。
阅读全文
相关推荐
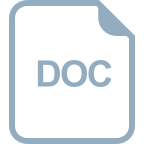
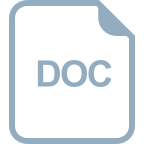
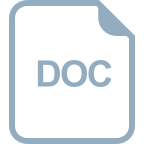




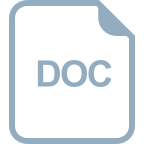
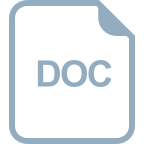
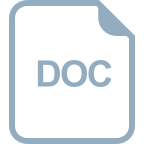
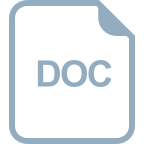
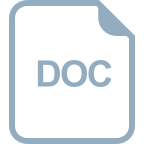






