把csv数据导入numpy
时间: 2023-10-20 11:05:58 浏览: 36
要将csv文件中的数据导入到numpy中,可以使用numpy的genfromtxt()函数。以下是一个示例代码:
```
import numpy as np
data = np.genfromtxt('data.csv', delimiter=',')
```
在这个示例中,我们使用了np.genfromtxt()函数来加载csv文件中的数据。delimiter参数指定了csv文件中的分隔符,这里使用了逗号作为分隔符。导入完成后,数据将存储在名为data的numpy数组中。
相关问题
numpy 读取csv数据
可以使用numpy中的genfromtxt函数来读取csv数据。具体步骤为:
1. 导入numpy模块,例如import numpy as np
2. 使用genfromtxt函数读取csv数据,例如data = np.genfromtxt('data.csv', delimiter=',')
其中,'data.csv'是csv文件的路径,delimiter=','表示以逗号为分隔符。
如果csv文件中含有字符串等非数字类型的数据,可以使用dtype参数指定相应的数据类型,例如:
data = np.genfromtxt('data.csv', delimiter=',', dtype=[('name', 'S10'), ('age', 'i4'), ('weight', 'f8')])
这样可以按照csv文件中每一列不同的数据类型读取数据。
numpy读取csv数据文件
可以使用numpy中的loadtxt函数来读取csv文件。具体步骤如下:
1. 导入numpy模块:import numpy as np
2. 使用loadtxt函数读取csv文件:data = np.loadtxt('filename.csv', delimiter=',', skiprows=1)
其中,filename.csv是要读取的csv文件名,delimiter是分隔符,skiprows表示跳过的行数,这里设为1是因为第一行通常是表头。
3. 读取后的数据可以直接使用,例如:print(data)
相关推荐
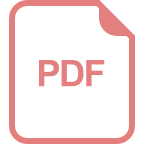
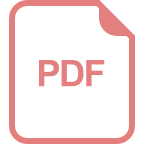
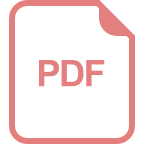
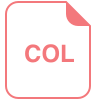
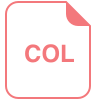
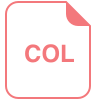









