yoloV8切分自己的数据集python代码源码
时间: 2024-09-13 21:16:56 浏览: 11
YOLOv8是You Only Look Once(YOLO)系列的最新版本,是一个流行的实时对象检测系统。在使用YOLOv8进行目标检测之前,通常需要准备并切分自己的数据集,以便训练和测试模型。以下是一个简化的示例,展示如何使用Python编写脚本来切分自己的数据集:
```python
import os
import shutil
from sklearn.model_selection import train_test_split
# 假设我们有以下结构的数据集,其中包含两个类别:dog和cat
# dataset/
# |-- dog/
# | |-- cat/
# |--- labels/
# |-- dog/
# |-- cat/
# 首先,我们需要列出所有的图片文件路径及其对应的标签
images_path = []
labels_path = []
# 假设我们的标签和图片都放在相应的子文件夹中
for label_folder in os.listdir('dataset/labels'):
for file in os.listdir(f'dataset/labels/{label_folder}'):
label_path = os.path.join('dataset/labels', label_folder, file)
image_path = os.path.join('dataset/images', label_folder, file.replace('.txt', '.jpg'))
images_path.append(image_path)
labels_path.append(label_path)
# 然后,我们将数据集切分为训练集、验证集和测试集
# 假设我们按照80%、10%、10%的比例进行切分
images_train, images_temp, labels_train, labels_temp = train_test_split(images_path, labels_path, test_size=0.2, random_state=42)
images_val, images_test, labels_val, labels_test = train_test_split(images_temp, labels_temp, test_size=0.5, random_state=42)
# 接下来,我们需要创建对应的目录结构
for dir in ['train', 'val', 'test']:
os.makedirs(f'dataset/{dir}/images', exist_ok=True)
os.makedirs(f'dataset/{dir}/labels', exist_ok=True)
# 然后,将切分后的数据复制到相应的目录中
def copy_to_directory(paths, dir_type):
for path in paths:
dir_name = 'images' if 'images' in path else 'labels'
dest_dir = f'dataset/train/{dir_name}' if dir_type == 'train' else f'dataset/val/{dir_name}' if dir_type == 'val' else f'dataset/test/{dir_name}'
shutil.copy(path, dest_dir)
# 调用函数将数据移动到对应的目录
copy_to_directory(images_train, 'train')
copy_to_directory(labels_train, 'train')
copy_to_directory(images_val, 'val')
copy_to_directory(labels_val, 'val')
copy_to_directory(images_test, 'test')
copy_to_directory(labels_test, 'test')
```
这段代码是一个非常基础的示例,它没有考虑YOLOv8数据集格式的具体要求,比如YOLO格式的标注文件中的具体内容。在实际使用中,你可能需要根据YOLOv8的具体要求对标注文件进行相应的格式化和处理。
相关推荐
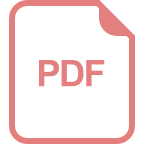
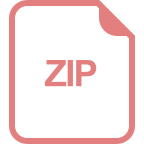
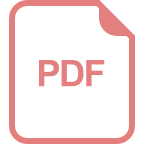














