编制时间片轮转调度算法,可自定义时间片的大小。 编制静态优先级调度算法,优先级高低可自定义。
时间: 2024-04-05 16:29:07 浏览: 12
好的,我明白了。对于时间片轮转调度算法,其基本思路是将所有进程按照到达时间排序,然后按照时间片进行轮流调度。具体步骤如下:
1. 将所有进程按照到达时间排序。
2. 设定时间片大小。
3. 将第一个进程放入就绪队列,并开始执行。
4. 当时间片用完时,将该进程放回队列尾部,并将下一个进程放入运行队列。
5. 重复步骤4,直到所有进程执行完毕。
对于静态优先级调度算法,其基本思路是通过设定不同的进程优先级,将优先级高的进程先执行。具体步骤如下:
1. 设定优先级范围。
2. 将所有进程按照优先级排序。
3. 将优先级最高的进程放入就绪队列,并开始执行。
4. 当该进程执行完毕后,将下一个优先级最高的进程放入运行队列。
5. 重复步骤4,直到所有进程执行完毕。
需要注意的是,静态优先级调度算法可能存在“饥饿”现象,即优先级低的进程可能永远得不到执行。因此,可以采用动态优先级调度算法来解决这个问题。
相关问题
编制时间片轮转调度算法,可自定义时间片的大小,编制静态优先级调度算法,优先级高低可自定义的c语言代码
时间片轮转调度算法 C 代码:
```c
#include <stdio.h>
#define MAX_PROCESS 10 // 最大进程数
#define MAX_TIME_SLOT 100 // 最大时间片数
#define MAX_ARRIVAL_TIME 100 // 最大到达时间
#define MAX_PRIORITY 10 // 最大优先级
struct process {
int pid; // 进程 ID
int arrival_time; // 到达时间
int burst_time; // 执行时间
int remaining_time; // 剩余执行时间
int priority; // 优先级
};
int main() {
int n, time_slot;
printf("请输入进程数:");
scanf("%d", &n);
struct process p[MAX_PROCESS];
int i;
// 输入进程信息
for (i = 0; i < n; i++) {
printf("请输入第 %d 个进程的到达时间、执行时间和优先级:", i+1);
scanf("%d%d%d", &p[i].arrival_time, &p[i].burst_time, &p[i].priority);
p[i].pid = i + 1;
p[i].remaining_time = p[i].burst_time;
}
printf("请输入时间片大小:");
scanf("%d", &time_slot);
int current_time = 0;
int completed_process = 0; // 已完成的进程数
int time_slot_count = 0; // 时间片计数器
while (completed_process < n) {
int selected_process = -1; // 选中的进程
int highest_priority = -1; // 最高优先级
// 选择优先级最高的进程
for (i = 0; i < n; i++) {
if (p[i].arrival_time <= current_time && p[i].remaining_time > 0 &&
(highest_priority == -1 || p[i].priority < highest_priority)) {
selected_process = i;
highest_priority = p[i].priority;
}
}
if (selected_process == -1) {
// 当前时间无进程可运行,跳过
current_time++;
continue;
}
// 运行进程
p[selected_process].remaining_time--;
time_slot_count++;
if (p[selected_process].remaining_time == 0) {
// 进程已完成
completed_process++;
printf("时间 %d : 进程 %d 完成\n", current_time, p[selected_process].pid);
} else if (time_slot_count == time_slot) {
// 时间片用完,切换进程
time_slot_count = 0;
printf("时间 %d : 进程 %d 时间片用完\n", current_time, p[selected_process].pid);
}
current_time++;
}
return 0;
}
```
静态优先级调度算法 C 代码:
```c
#include <stdio.h>
#define MAX_PROCESS 10 // 最大进程数
#define MAX_ARRIVAL_TIME 100 // 最大到达时间
#define MAX_PRIORITY 10 // 最大优先级
struct process {
int pid; // 进程 ID
int arrival_time; // 到达时间
int burst_time; // 执行时间
int remaining_time; // 剩余执行时间
int priority; // 优先级
};
int main() {
int n;
printf("请输入进程数:");
scanf("%d", &n);
struct process p[MAX_PROCESS];
int i;
// 输入进程信息
for (i = 0; i < n; i++) {
printf("请输入第 %d 个进程的到达时间、执行时间和优先级:", i+1);
scanf("%d%d%d", &p[i].arrival_time, &p[i].burst_time, &p[i].priority);
p[i].pid = i + 1;
p[i].remaining_time = p[i].burst_time;
}
// 按优先级排序
for (i = 0; i < n - 1; i++) {
int j, max_priority_index = i;
for (j = i + 1; j < n; j++) {
if (p[j].priority < p[max_priority_index].priority) {
max_priority_index = j;
}
}
if (i != max_priority_index) {
struct process temp = p[i];
p[i] = p[max_priority_index];
p[max_priority_index] = temp;
}
}
int current_time = 0;
int completed_process = 0; // 已完成的进程数
while (completed_process < n) {
int selected_process = -1; // 选中的进程
// 选择优先级最高的进程
for (i = 0; i < n; i++) {
if (p[i].arrival_time <= current_time && p[i].remaining_time > 0) {
selected_process = i;
break;
}
}
if (selected_process == -1) {
// 当前时间无进程可运行,跳过
current_time++;
continue;
}
// 运行进程
p[selected_process].remaining_time--;
if (p[selected_process].remaining_time == 0) {
// 进程已完成
completed_process++;
printf("时间 %d : 进程 %d 完成\n", current_time, p[selected_process].pid);
}
current_time++;
}
return 0;
}
```
写一个编制时间片轮转调度算法,可自定义时间片的大小 ,编制静态优先级调度算法,优先级高低可自定义的代码
好的,以下是Python 3中编制时间片轮转调度算法的代码和编制静态优先级调度算法的代码,都可以自定义时间片大小和优先级高低:
时间片轮转调度算法:
```python
def time_slice_rr(processes, time_slice):
n = len(processes)
queue = [] # 进程队列
current_time = 0 # 当前时间
total_time = 0 # 运行总时间
index = 0 # 当前执行的进程在队列中的位置
# 将所有进程按照到达时间顺序加入队列
for process in processes:
queue.append(process)
while True:
# 选择下一个要执行的进程
if not queue:
break
current_process = queue[index]
# 执行进程
if current_process[1] > time_slice:
current_process[1] -= time_slice
current_time += time_slice
total_time += time_slice
# 将该进程放回队列末尾
queue.append(queue.pop(index))
else:
current_time += current_process[1]
total_time += current_process[1]
current_process[1] = 0
current_process.append(current_time) # 记录结束时间
queue.pop(index)
# 更新 index
index += 1
if index >= len(queue):
index = 0
return processes
```
静态优先级调度算法:
```python
def static_priority(processes, priority_list):
n = len(processes)
queue = [] # 进程队列
current_time = 0 # 当前时间
total_time = 0 # 运行总时间
# 将所有进程按照优先级顺序加入队列
for i in range(n):
priority = priority_list[i]
queue.append(processes[priority])
while True:
# 选择优先级最高的进程
if not queue:
break
current_process = queue[0]
# 执行进程
current_time += current_process[1]
total_time += current_process[1]
current_process[1] = 0
current_process.append(current_time) # 记录结束时间
queue.pop(0)
return processes
```
以上代码仅供参考,具体实现还需要根据实际需求进行修改。
相关推荐
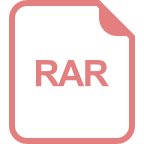
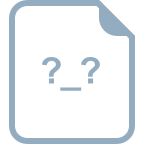












