用Java编写 先写出正常运行时的代码:电脑开机后上课 分析电脑会出现的问题,如:蓝屏,冒烟 此时需要自定义两个“蓝屏,冒烟“”自定义异常,封装成对象。 蓝屏后,电脑重启,之后继续上课 电脑冒烟后,出现了老师的问题,不能上课了,可以先做练习,毕老师将问题抛给校长。此时抛出的问题不再是电脑冒烟,而应该是老师不能上课了 问题抛给校长后,校长catch后给出解决方案
时间: 2023-09-29 09:07:18 浏览: 33
以下是可能的实现:
```java
public class Computer {
private boolean isOn;
public void turnOn() {
// 电脑正常启动
isOn = true;
System.out.println("电脑启动成功,可以上课了");
}
public void blueScreen() throws BlueScreenException {
// 电脑蓝屏
isOn = false;
throw new BlueScreenException("电脑蓝屏了");
}
public void restart() {
// 电脑重启
isOn = true;
System.out.println("电脑重启成功,可以继续上课");
}
public void smoke() throws SmokeException {
// 电脑冒烟
isOn = false;
throw new SmokeException("电脑冒烟了");
}
}
class BlueScreenException extends Exception {
public BlueScreenException(String message) {
super(message);
}
}
class SmokeException extends Exception {
public SmokeException(String message) {
super(message);
}
}
class Teacher {
public void teach(Computer computer) {
try {
computer.turnOn();
computer.blueScreen();
computer.restart();
computer.smoke();
} catch (BlueScreenException e) {
System.out.println(e.getMessage());
computer.restart();
} catch (SmokeException e) {
System.out.println(e.getMessage());
System.out.println("老师不能上课了,可以先做练习");
throw new TeacherCannotTeachException("老师不能上课了");
}
}
}
class TeacherCannotTeachException extends RuntimeException {
public TeacherCannotTeachException(String message) {
super(message);
}
}
class Principal {
public void handleException(Exception e) {
if (e instanceof TeacherCannotTeachException) {
System.out.println("校长:老师不能上课了,我会想办法解决的");
} else {
System.out.println("校长:出现了其他问题,需要进一步处理");
}
}
}
public class Main {
public static void main(String[] args) {
Computer computer = new Computer();
Teacher teacher = new Teacher();
Principal principal = new Principal();
try {
teacher.teach(computer);
} catch (Exception e) {
principal.handleException(e);
}
}
}
```
在这个例子中,我们定义了两个自定义异常类 `BlueScreenException` 和 `SmokeException` 来表示电脑可能出现的两种问题,将它们封装成异常对象抛出。当电脑出现蓝屏时,我们会捕获 `BlueScreenException` 异常并尝试重启电脑;当电脑冒烟时,我们会捕获 `SmokeException` 异常并将问题抛给校长。当校长捕获到 `TeacherCannotTeachException` 异常时,会给出解决方案。
相关推荐
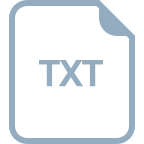
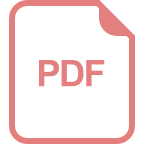














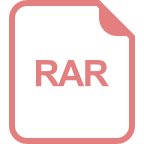